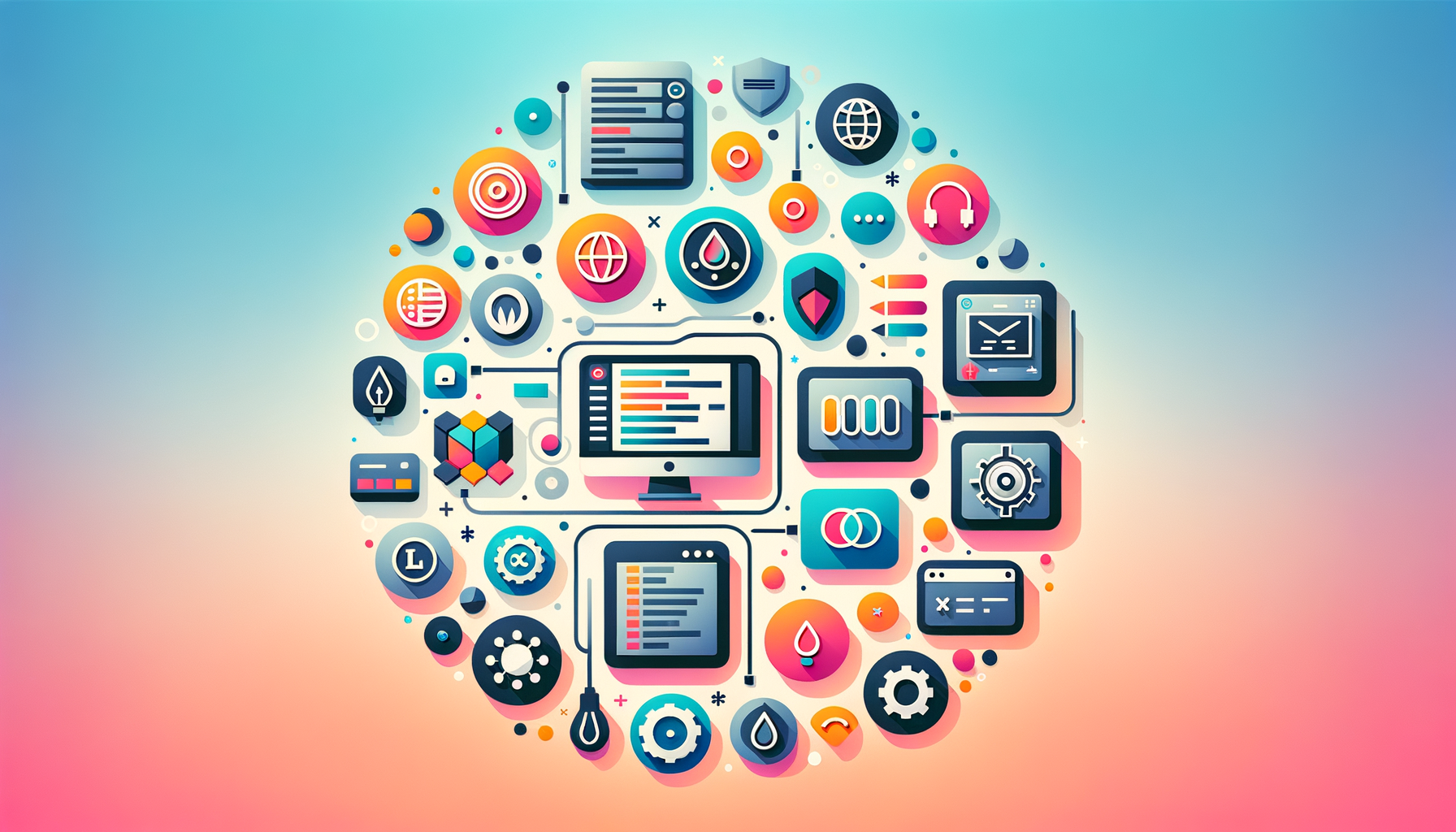
## Embarking on WordPress Plugin Development: A Comprehensive Guide
Creating a custom WordPress plugin is an exciting and rewarding project that can significantly enhance the functionality of your WordPress site. Whether you are a seasoned developer or just starting out, this guide will walk you through the entire process, from conceptualizing your plugin to deploying it.
Setting Up Your Development Environment
Before you dive into coding, it’s crucial to set up a robust development environment. This ensures you can test and develop your plugin in a controlled and safe space without affecting your live site.
To set up a local WordPress installation, you can use tools like MAMP, XAMPP, or Local by Flywheel. These tools make it easy to run WordPress on your computer, allowing you to develop and test your plugin safely.
Here’s a step-by-step guide to setting up a local environment:
- Download and Install: Download the tool of your choice (e.g., Local by Flywheel) and follow the installation instructions.
- Create a New Site: Use the tool to create a new WordPress site on your local machine.
- Access Your Site: You can now access your local WordPress site through the tool’s interface.
Defining Your Plugin Idea
Every great plugin starts with a unique idea. Identify a problem that needs solving or an enhancement to existing WordPress functionality. The best plugins offer solutions that haven’t been explored yet.
- Research Existing Plugins: Check the WordPress plugin directory to see if a similar plugin already exists. If it does, think about how you can improve upon it or offer something unique.
- User Feedback: Consider gathering feedback from users to understand what features they need or what problems they face.
Planning Your Plugin
Planning is crucial for successful plugin development. Here are some steps to help you plan your plugin:
- Outline Features: Make a list of the features your plugin will have. Consider how these features will interact with each other and with the core WordPress functionality.
- Structure Your Code: Think about the structure of your plugin. Will it be a simple plugin with a single PHP file, or will it require multiple files and directories? For more complex plugins, you might need separate subdirectories for different types of files.
- User Interaction: Ensure the user experience is intuitive and rewarding. Consider how users will navigate through your plugin’s features.
Creating Your Plugin File
Creating your own plugin starts with writing a plugin header. This header is essential as it tells WordPress the name of your plugin, who created it, and what it does.
Here’s an example of what a plugin header might look like:
<?php
/**
* Plugin Name: My Awesome Plugin
* Plugin URI: http://example.com
* Description: This plugin adds awesomeness to your site.
* Version: 1.0
* Author: Your Name
* Author URI: http://example.com
*/
?>
This code goes at the very beginning of your plugin file and provides essential information to WordPress.
Writing Basic PHP Functions
Now, it’s time to write the PHP functions that will power your plugin. Here’s a simple example of a plugin that adds a special greeting to your posts:
function my_greeting() {
echo 'Welcome to my website';
}
add_action('the_content', 'my_greeting');
This code creates a function called my_greeting
that echoes a welcome message. The add_action
line tells WordPress to add this greeting to the content of your posts.
For more complex plugins, you can use various WordPress hooks, such as actions and filters, to integrate your plugin seamlessly with core functionality. You can consult resources like the WordPress Plugin Handbook and Adam Brown’s WordPress hooks list.
Utilizing Hooks and Shortcodes
Hooks are a powerful way to extend WordPress functionality without modifying the core files. Here’s how you can use hooks and shortcodes in your plugin:
- Actions: Use
add_action
to execute your functions at specific points in WordPress’s execution flow. - Filters: Use
add_filter
to modify data before it is displayed or saved. - Shortcodes: Use
add_shortcode
to create custom shortcodes that users can use in their posts and pages.
Creating Custom Settings for Your Plugin
As your plugin grows, you may want to give users the ability to customize its behavior. Here’s a simple example of how to add a settings page:
function my_plugin_settings_page() {
add_options_page(
'My Plugin Settings',
'My Plugin',
'manage_options',
'my-plugin-settings',
'my_plugin_settings_callback'
);
}
add_action('admin_menu', 'my_plugin_settings_page');
function my_plugin_settings_callback() {
// Your settings page content here
}
This code adds a new settings page to the WordPress admin area where users can configure your plugin.
Uploading and Activating Your Plugin
Once you’ve developed and tested your plugin, it’s time to upload it to your WordPress site.
- Package Your Plugin: Create a ZIP file of your plugin folder. On Mac, right-click on the folder and select ‘Compress’, while on Windows, right-click and select ‘Send to > Compressed (zipped) folder’.
- Upload the Plugin: Go to the WordPress admin area, navigate to Plugins > Add New, and click on the ‘Upload Plugin’ button. Select the ZIP file and click ‘Install Now’.
- Activate the Plugin: After installation, activate the plugin from the Plugins page.
Testing Your Plugin
Testing is a critical step in plugin development. Here are some tips for testing your plugin:
- Local Testing: Test your plugin on your local development environment before deploying it to a live site.
- Staging Environment: Use a staging environment to test your plugin in a setting that mimics your live site.
- User Testing: Get feedback from users to ensure your plugin works as expected and is user-friendly.
Distributing Your Plugin
If you want to share your plugin with the wider WordPress community, you can submit it to the WordPress plugin directory.
- Create a Read Me File: Write a
readme.txt
file that explains what your plugin does, its features, and how to use it. - Submit to WordPress.org: Log in to your WordPress.org account, go to the Add Your Plugin page, and upload your plugin’s ZIP file. Ensure your plugin complies with the Detailed Plugin Guidelines before submission.
Maintaining Your Plugin
After deployment, maintaining your plugin is crucial to ensure it continues to work smoothly with future WordPress updates.
- Regular Updates: Keep your plugin updated to ensure compatibility with the latest version of WordPress.
- User Feedback: Continuously gather feedback from users to improve and add new features.
- Security: Ensure your plugin follows best security practices to protect against vulnerabilities.
For more resources on maintaining your plugin, you can refer to the WordPress Plugin Handbook and WPSeek.
Conclusion and Next Steps
Creating a custom WordPress plugin is a rewarding experience that can significantly enhance the functionality of your WordPress site. By following these steps, you can develop a plugin that is both functional and user-friendly.
If you need further assistance or want to develop a custom WordPress plugin for your business, consider reaching out to a professional agency like Belov Digital Agency. Our team of experts can help you from concept to deployment, ensuring your plugin meets the highest standards of quality and performance.
For more information on how we can help, visit our Contact Us page or check out our other blog posts on WordPress development.
Additionally, if you’re looking for reliable hosting to support your WordPress site and plugins, consider using Kinsta, a top-tier hosting solution that ensures high performance and security.