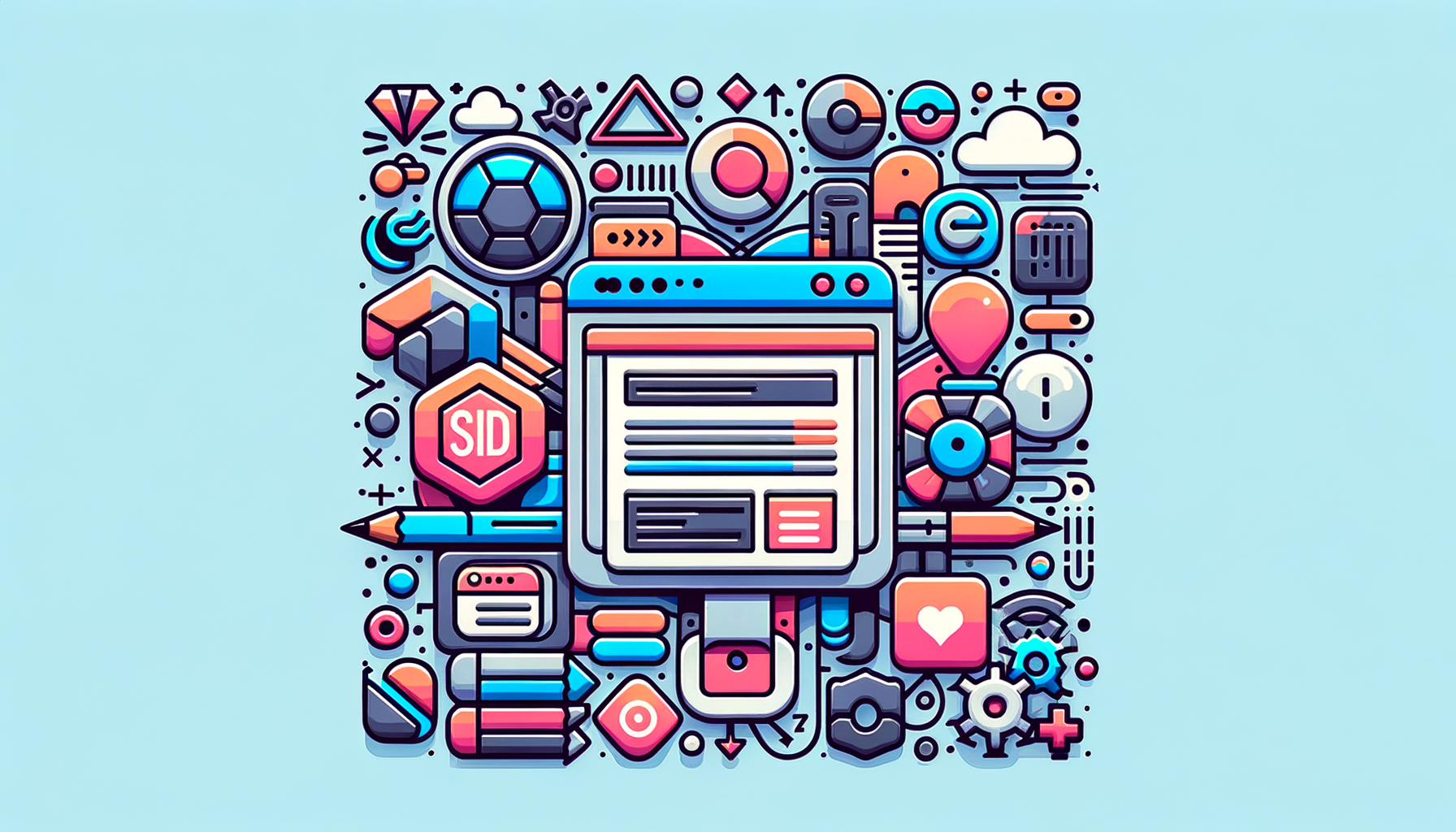
Enhancing Client Websites with Custom Gutenberg Blocks
In the ever-evolving landscape of web development, the WordPress block editor, also known as Gutenberg, has revolutionized the way content is created and managed. For agencies like Belov Digital, creating custom Gutenberg blocks is a powerful way to offer clients enhanced content flexibility and a more streamlined user experience. Here’s a comprehensive guide on how to create custom Gutenberg blocks, complete with real-world examples and actionable steps.
Understanding the Block Editor
Before diving into the creation of custom blocks, it’s essential to understand the basics of the Gutenberg block editor. Introduced in WordPress 5.0, Gutenberg replaces the traditional TinyMCE editor with a block-based system. This allows users to build content using individual blocks for text, images, videos, and more, making content creation more intuitive and flexible.
Tools and Methods for Creating Custom Blocks
There are several methods and tools available for creating custom Gutenberg blocks, each catering to different levels of development expertise.
Using Official Tools: @wordpress/create-block
One of the most straightforward ways to create a custom Gutenberg block is by using the official @wordpress/create-block
tool. This zero-configuration tool generates all the necessary files and folders for your block plugin, including PHP, JS, and SCSS code. Here’s how you can get started:
npx @wordpress/create-block my-custom-block
This command sets up a starter block with a modern build setup, inspired by create-react-app
. You can then navigate to the newly created directory and run npm start
to start the development server.
Using Third-Party Tools: create-guten-block
Another popular tool is create-guten-block
, which is also based on create-react-app
and provides a quick way to generate your first block plugin without configuring React, Webpack, or other dependencies.
npx create-guten-block my-custom-block
This tool provides everything you need to create a modern WordPress plugin, including the necessary files and folders. After setting up the project, you can activate the plugin in your WordPress dashboard and start using your custom block.
Step-by-Step Guide to Creating a Custom Block
Here’s a detailed step-by-step guide to creating a custom Gutenberg block, using both plugin-based and manual methods.
Step 1: Setting Up the Block Plugin
To manually create a custom block, you need to set up a plugin that will enqueue your block scripts and add them to the editor. Here’s how you can do it:
- Access your website via SFTP and navigate to the
wp-content/plugins
directory. - Create a new folder for your plugin (e.g.,
my-custom-block
). - Inside this folder, create a
plugin.php
file and add the necessary code to register your block:
<?php
/*
Plugin Name: My Custom Block
Description: A custom block for WordPress.
Version: 1.0
Author: Your Name
Author URI: https://yourwebsite.com
*/
function register_my_custom_block() {
wp_enqueue_script(
'my-custom-block',
plugins_url( 'block.js', __FILE__ ),
array( 'wp-blocks', 'wp-element' )
);
register_block_type( 'my-custom-block/my-custom-block', array(
'editor_script' => 'my-custom-block',
) );
}
add_action( 'enqueue_block_assets', 'register_my_custom_block' );
Create a block.js
file in the same directory and add the JavaScript code to define your block using React:
import { registerBlockType } from '@wordpress/blocks';
import { RichText, InspectorControls, ColorPalette } from '@wordpress/block-editor';
import { Button } from '@wordpress/components';
registerBlockType( 'my-custom-block/my-custom-block', {
title: 'My Custom Block',
icon: 'admin-users',
category: 'common',
attributes: {
content: { type: 'string' },
backgroundColor: { type: 'string' },
},
edit: EditComponent,
save: SaveComponent,
} );
function EditComponent( props ) {
const { attributes, setAttributes } = props;
const { content, backgroundColor } = attributes;
return (
<div style={ { backgroundColor: backgroundColor } }>
<RichText
value={ content }
onChange={ ( newContent ) => setAttributes( { content: newContent } ) }
placeholder="Enter your text here"
/>
<InspectorControls>
<ColorPalette
value={ backgroundColor }
onChange={ ( newBackgroundColor ) => setAttributes( { backgroundColor: newBackgroundColor } ) }
/>
</InspectorControls>
</div>
);
}
function SaveComponent( props ) {
const { attributes } = props;
const { content, backgroundColor } = attributes;
return (
<div style={ { backgroundColor: backgroundColor } }>
<p>{ content }</p>
</div>
);
}
Step 2: Registering and Configuring the Block
After setting up the plugin and defining your block, you need to register it and configure its attributes. Here’s an example of how you can register a block and configure its attributes:
- Register the block in your
plugin.php
file as shown above. - Define the block attributes and the edit and save components in your
block.js
file.
Step 3: Creating the Block Template
The block template determines how the information entered into the block is displayed on your website. You can use HTML, CSS, or PHP to create the template.
Using the Built-in Template Editor: You can use the built-in template editor to create and style your block. For example, for a testimonial block:
<div class="testimonial-item">
<img src="{{reviewer-image}}" class="reviewer-image">
<h4 class="reviewer-name">{{reviewer-name}}</h4>
<div class="testimonial-text">{{testimonial-text}}</div>
</div>
You can then switch to the CSS tab to style your block output markup.
Manual Template Creation: If your block requires PHP to run in the background, you need to manually create a block template file and upload it to your theme folder. Here’s an example of how you can create a block.php
file:
<?php
function block_field() {
// Fetch and display the block data
$reviewerImage = get_field( 'reviewer-image' );
$reviewerName = get_field( 'reviewer-name' );
$testimonialText = get_field( 'testimonial-text' );
?>
<div class="testimonial-item">
<img src="<?php echo $reviewerImage; ?>" class="reviewer-image">
<h4 class="reviewer-name"><?php echo $reviewerName; ?></h4>
<div class="testimonial-text"><?php echo $testimonialText; ?></div>
</div>
<?php
}
Step 4: Using the Custom Block
After creating and configuring your custom block, you can use it on your WordPress website.
- Navigate to the page or post where you want to add the block.
- Click the “Add Block” button and search for your custom block.
- Drag and drop the block into the editor and input the content you want to display.
Real-World Examples and Case Studies
Testimonial Block
Creating a testimonial block is a common use case for custom Gutenberg blocks. Here’s how you can create one:
- Use the
Genesis Custom Blocks
plugin or manual methods to create a block with fields for the reviewer’s image, name, and testimonial text. - Style the block using CSS to match your website’s design.
- Use the block on multiple pages or posts to display testimonials from clients or customers.
Contact Information Block
Another useful block is a contact information block that includes fields for the company name, phone number, and address.
- Create the block using
@wordpress/create-block
orcreate-guten-block
. - Define the attributes and edit/save components to handle the contact information.
- Use the block on your website’s contact page or footer to display the contact information consistently.
Best Practices and Considerations
Testing in Staging Environments
When creating and testing custom Gutenberg blocks, it’s crucial to do so in a staging or local environment to avoid disrupting your live website. Tools like Local by WP Engine can help you set up a local development environment quickly.
Hosting and Performance
Ensure that your hosting provider supports the latest versions of WordPress and Gutenberg. High-performance hosting solutions like Kinsta can help optimize the performance of your custom blocks and overall website.
Maintenance and Updates
Regularly update your custom blocks to ensure compatibility with the latest WordPress and Gutenberg updates. This can be done by updating the plugin files and testing the blocks in a staging environment before deploying them to your live site.
Conclusion and Next Steps
Creating custom Gutenberg blocks offers a powerful way to enhance the content flexibility and user experience of client websites. By using the right tools and following best practices, you can develop blocks that are both functional and visually appealing.
If you’re looking to take your WordPress development skills to the next level or need assistance with creating custom Gutenberg blocks, consider reaching out to Belov Digital Agency for expert guidance and support.
Remember, the key to successful custom block development is thorough testing, regular maintenance, and a deep understanding of the Gutenberg block editor. With these insights, you can create custom blocks that elevate your clients’ websites and provide a superior user experience.