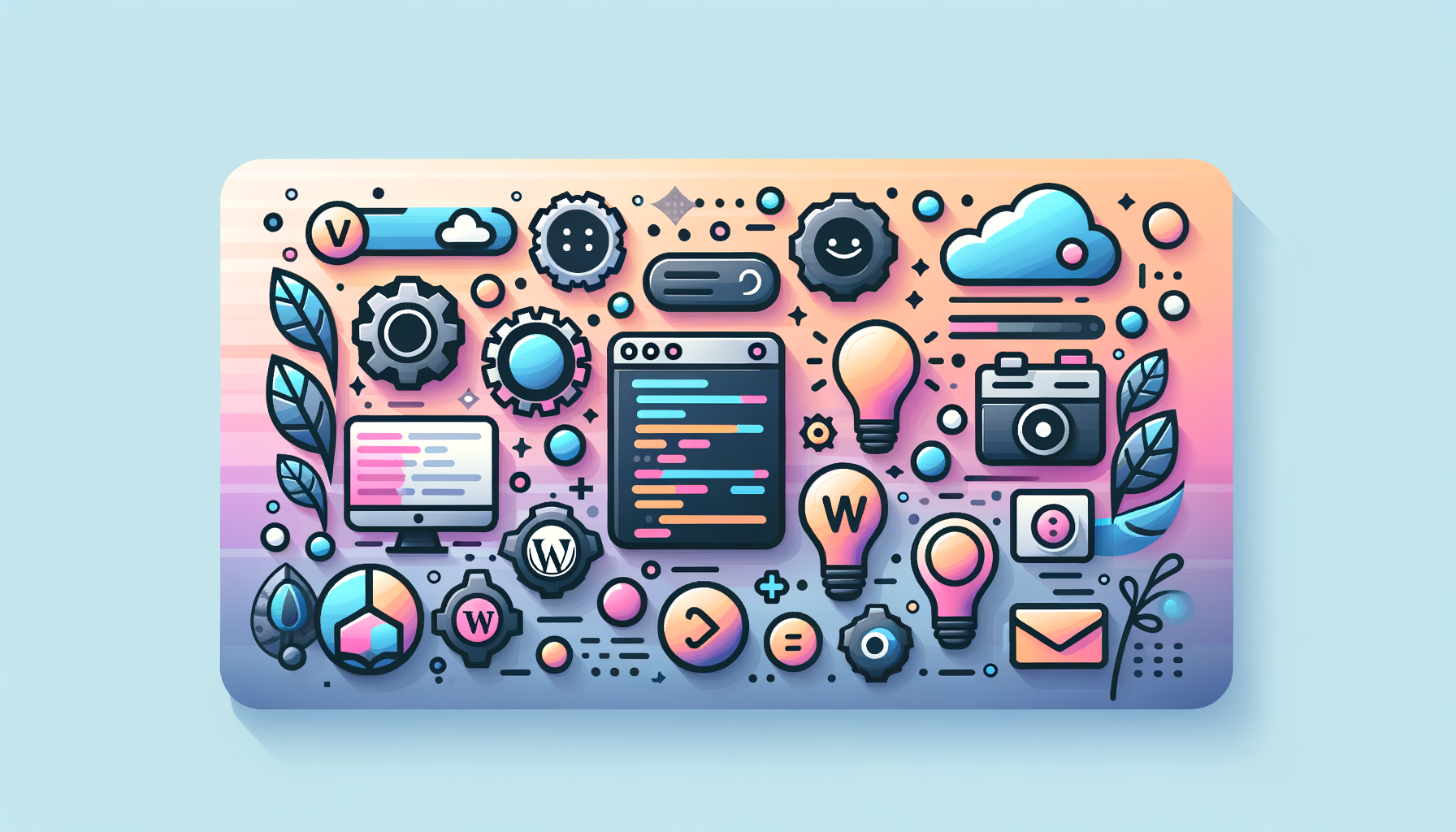
Tailoring WordPress Functionality for Agency Needs
When it comes to enhancing the capabilities of a WordPress website, custom plugin development stands out as a powerful and flexible solution. For digital agencies, creating custom WordPress plugins can be a game-changer, allowing them to provide tailored solutions that meet the unique needs of their clients. Here’s a comprehensive guide on how to create custom WordPress plugins for agency-specific functionality.
Identifying the Need for Custom Plugins
Before diving into the development process, it’s crucial to identify why a custom plugin is necessary. Custom plugins offer several advantages over using existing plugins from the WordPress repository:
- Extend Functionality: Custom plugins can add features that are not available in the core WordPress installation or existing plugins. For example, integrating a client’s WordPress site with their ERP (Enterprise Resource Planning) or CRM (Customer Relationship Management) systems.
- Efficient Customization: Custom plugins allow for tailored solutions that align perfectly with the client’s branding and business needs, avoiding the limitations of generic plugins.
- Internal Features: You can create internal tools or features specific to your business operations, such as custom post types, advanced search and filtering options, or even a secure patient portal for healthcare websites.
Planning Your Plugin
Planning is a critical phase in plugin development. Here are some steps to help you plan your plugin effectively:
Define Your Plugin Idea
Every great plugin starts with a unique idea. Identify a problem that needs solving or an enhancement to existing WordPress functionality. Research existing plugins to see if a similar solution already exists and think about how you can improve upon it.
Outline Features
Make a list of the features your plugin will have. Consider how these features will interact with each other and with the core WordPress functionality. For instance, if you’re developing a plugin for an e-commerce site, you might need to integrate a custom payment gateway or shipping integration.
Structure Your Code
Think about the structure of your plugin. Will it be a simple plugin with a single PHP file, or will it require multiple files and directories? For more complex plugins, you might need separate subdirectories for different types of files.
Creating Your Plugin File
Creating your own plugin starts with writing a plugin header. This header is essential as it tells WordPress the name of your plugin, who created it, and what it does.
<?php
/**
* Plugin Name: My Awesome Plugin
* Plugin URI: http://example.com
* Description: This plugin adds awesomeness to your site.
* Version: 1.0
* Author: Your Name
* Author URI: http://example.com
*/
?>
This code goes at the very beginning of your plugin file and provides essential information to WordPress.
Writing Basic PHP Functions
Now, it’s time to write the PHP functions that will power your plugin. Here’s a simple example of a plugin that adds a special greeting to your posts:
function my_greeting() {
echo 'Welcome to my website';
}
add_action('the_content', 'my_greeting');
This code creates a function called my_greeting
that echoes a welcome message. The add_action
line tells WordPress to add this greeting to the content of your posts.
Utilizing Hooks and Shortcodes
Hooks are a powerful way to extend WordPress functionality without modifying the core files. Here’s how you can use hooks and shortcodes in your plugin:
- Actions: Use
add_action
to execute your functions at specific points in WordPress’s execution flow. - Filters: Use
add_filter
to modify data before it is displayed or saved. - Shortcodes: Use
add_shortcode
to create custom shortcodes that users can use in their posts and pages.
Creating Custom Settings for Your Plugin
As your plugin grows, you may want to give users the ability to customize its behavior. Here’s a simple example of how to add a settings page:
function my_plugin_settings_page() {
add_options_page(
'My Plugin Settings',
'My Plugin',
'manage_options',
'my-plugin-settings',
'my_plugin_settings_callback'
);
}
add_action('admin_menu', 'my_plugin_settings_page');
function my_plugin_settings_callback() {
// Your settings page content here
}
This code adds a new settings page to the WordPress admin area where users can configure your plugin.
Managing Permissions Using Capabilities
WordPress has a robust role and capability system that allows you to control user permissions. This is essential for secure plugin management and customization. Here’s an example of how to add a custom capability:
function add_custom_capability() {
$role = get_role('administrator');
$role->add_cap('manage_custom_feature');
}
add_action('admin_init', 'add_custom_capability');
// Checking for a capability
if (current_user_can('manage_custom_feature')) {
// User can manage custom feature
}
This code adds a custom capability and checks if the current user has that capability.
Hosting and Managing Your Plugin
For businesses needing to manage their custom plugins internally, hosting plugins on GitHub can be an excellent solution. GitHub provides a platform for version control and collaborative development, making it easier to manage changes and contributions from multiple developers.
- Create a Repository: Set up a new repository on GitHub.
- Push Your Plugin: Commit your plugin code and push it to the repository.
- Install via Composer: Use Composer to include your plugin in your WordPress site, ensuring easy updates and dependency management.
Real-World Examples and Case Studies
Custom plugins can be incredibly versatile and are used in various industries. Here are a few examples:
- E-commerce Websites: Custom plugins can be used to add a custom payment gateway, shipping integration, or a particular type of product display and filtering.
- Real Estate Websites: Plugins can integrate with third-party MLS listings or allow real estate agents to upload property listings with customized search options.
- Healthcare Websites: Custom plugins can be developed for secure patient portals, prescription refills, and appointment scheduling.
Best Practices and Advanced Techniques
To ensure your plugins are robust and reliable, follow these best practices:
- Prefix Your Functions: Always prefix your function names to avoid conflicts with other plugins.
- Use GitHub Actions: Automate your plugin testing and deployment processes using GitHub Actions to save time and reduce errors.
- Optimize Performance: Conduct thorough testing of your plugins across various devices and browsers to guarantee optimal performance and compatibility.
Conclusion and Next Steps
Creating custom WordPress plugins is a powerful way to customize and extend the functionality of WordPress websites. By following the steps outlined above and incorporating advanced techniques, you can develop plugins that enhance user experience, meet specific business needs, and integrate seamlessly with other systems.
If you need further assistance or want to develop a custom WordPress plugin for your business, consider reaching out to a professional agency like Belov Digital Agency. Our team of experts can help you from concept to deployment, ensuring your plugin meets the highest standards of quality and performance.
For reliable hosting to support your WordPress site and plugins, consider using Kinsta, a top-tier hosting solution that ensures high performance and security.
For more information on how we can help, visit our Contact Us page or check out our other blog posts on WordPress development, such as Creating a Custom WordPress Plugin: From Concept to Deployment.
By investing in custom WordPress plugin development, you can provide tailored solutions that elevate your website’s functionality and user experience, setting you apart in the digital landscape.