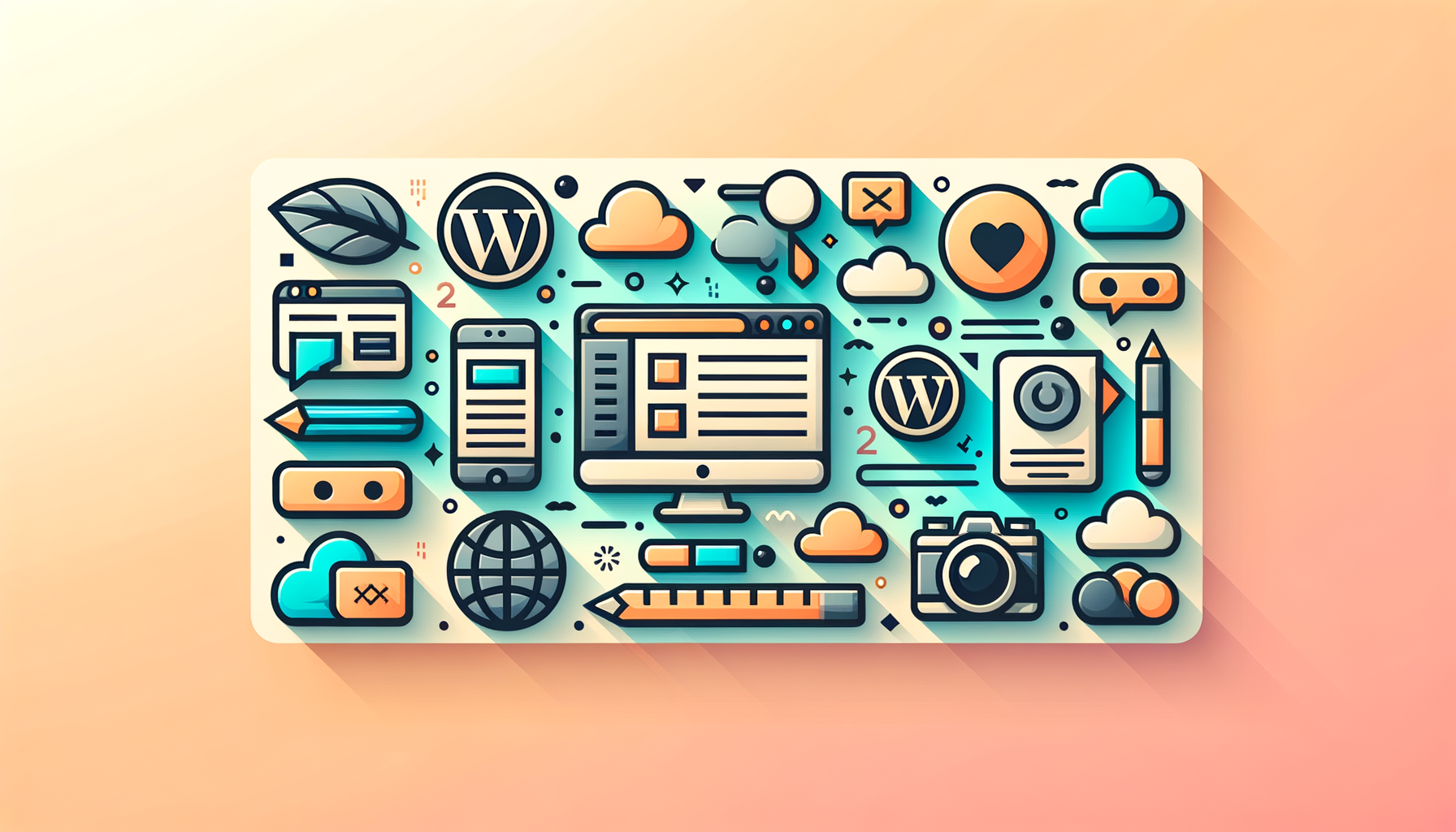
Unlocking the Power of Customization: A Comprehensive Guide to Creating Custom WordPress Widgets
Custom WordPress widgets are a powerful tool for enhancing the functionality and appearance of your website. Whether you’re looking to add a unique feature, improve user engagement, or simply personalize your site’s layout, creating custom widgets can be a game-changer. In this guide, we’ll walk you through the process of developing custom WordPress widgets, providing you with the knowledge and tools you need to take your website to the next level.
Understanding WordPress Widgets
Before diving into the creation process, it’s essential to understand what WordPress widgets are and how they work. Widgets are pieces of code that can be added to widget-ready areas of your website, such as sidebars, headers, and footers. They can display various types of content, from simple text and images to complex data feeds and interactive forms.
Determining the Purpose of Your Widget
Before you start coding, it’s crucial to determine the purpose and functionality of your widget. This involves planning where the widget will be placed and what unique features it will offer. For instance, if you’re creating a widget to display recent posts, you’ll want to place it in a prominent sidebar area where users are likely to see it.
Step-by-Step Guide to Creating Custom WordPress Widgets
Step 1: Setting Up Your Environment
To create a custom widget, you’ll need a local development environment. You can set up WordPress locally using tools like MAMP for Mac or WAMP for Windows. This allows you to test and develop your widget without affecting your live site.
Step 2: Extending the WP_Widget Class
The core of creating a custom widget involves extending the WP_Widget
class. This class provides the necessary methods to define and display your widget. Here are the four essential methods you’ll need to implement:
- __construct(): This constructor function defines your widget’s parameters and sets up its basic information.
- widget(): This method contains the output of the widget, which is what users will see on the front end of your site.
- form(): This method determines the widget settings displayed in the WordPress admin area.
- update(): This method updates the widget settings when new settings are saved in the admin area.
Example Code
class My_Widget extends WP_Widget {
function __construct() {
parent::__construct(
'my_widget', // Base ID
__('My Widget', 'text_domain'), // Name
array('description' => __('A custom widget', 'text_domain'),) // Args
);
}
function widget($args, $instance) {
// Output the widget content
echo $args['before_widget'];
if (!empty($instance['title'])) {
echo $args['before_title'] . apply_filters('widget_title', $instance['title']) . $args['after_title'];
}
// Your widget content here
echo "Hello, World!";
echo $args['after_widget'];
}
function form($instance) {
// Widget admin form
if (isset($instance['title'])) {
$title = $instance['title'];
} else {
$title = __('New title', 'text_domain');
}
?>
<?php
}
function update($new_instance, $old_instance) {
// Update the widget settings
$instance = array();
if (!empty($new_instance['title'])) {
$instance['title'] = strip_tags($new_instance['title']);
}
return $instance;
}
}
Step 3: Registering Your Widget
Once you’ve defined your widget class, you need to register it with WordPress. This is done using the widgets_init
action hook.
add_action('widgets_init', function(){
register_widget('My_Widget');
});
Step 4: Turning Your Widget into a Custom Plugin
If you want your widget to be theme-independent and reusable across multiple sites, consider turning it into a custom plugin. This involves creating a plugin file and adding the necessary header information.
Real-World Examples and Case Studies
Let’s look at a few examples of how custom widgets can be used in real-world scenarios:
- Recent Posts Widget: You can create a widget that displays a list of recent posts, complete with thumbnails and excerpts. This can be particularly useful for blogs and news sites.
- Social Media Feed Widget: A custom widget can be designed to display social media feeds, such as Twitter or Instagram updates, directly on your website.
- Contact Form Widget: For businesses, a custom contact form widget can be a valuable addition, allowing visitors to easily get in touch without navigating away from the main content.
Best Practices and Tools
When developing custom widgets, it’s important to follow best practices to ensure compatibility and performance:
- Use a Child Theme: If you’re adding custom code to your theme’s
functions.php
file, use a child theme to prevent losing your changes during theme updates. - Backup Your Site: Always create a full backup of your WordPress site before making significant changes.
- Use Code Snippets Plugin: The Code Snippets plugin can be a useful tool for adding and managing custom code snippets, including widget code.
Customizing Widget Appearance
Once you’ve created your custom widget, you can further enhance its appearance by adding custom styles. You can do this by visiting the Appearance » Customize page and adding CSS rules to target your widget’s specific CSS class.
Conclusion and Next Steps
Creating custom WordPress widgets is a powerful way to extend the functionality of your website. By following the steps outlined in this guide, you can develop widgets that meet your specific needs and enhance user engagement. If you need further assistance or have questions about WordPress development, feel free to Contact Us at Belov Digital Agency.
For more resources on WordPress development, check out our other blog posts, such as How to Optimize WordPress Performance and Best WordPress Hosting Options.
Remember, with the right tools and knowledge, you can unlock the full potential of your WordPress site. Consider hosting your site with reliable providers like Kinsta to ensure optimal performance and security.
Happy coding, and don’t hesitate to reach out if you need any help along the way!