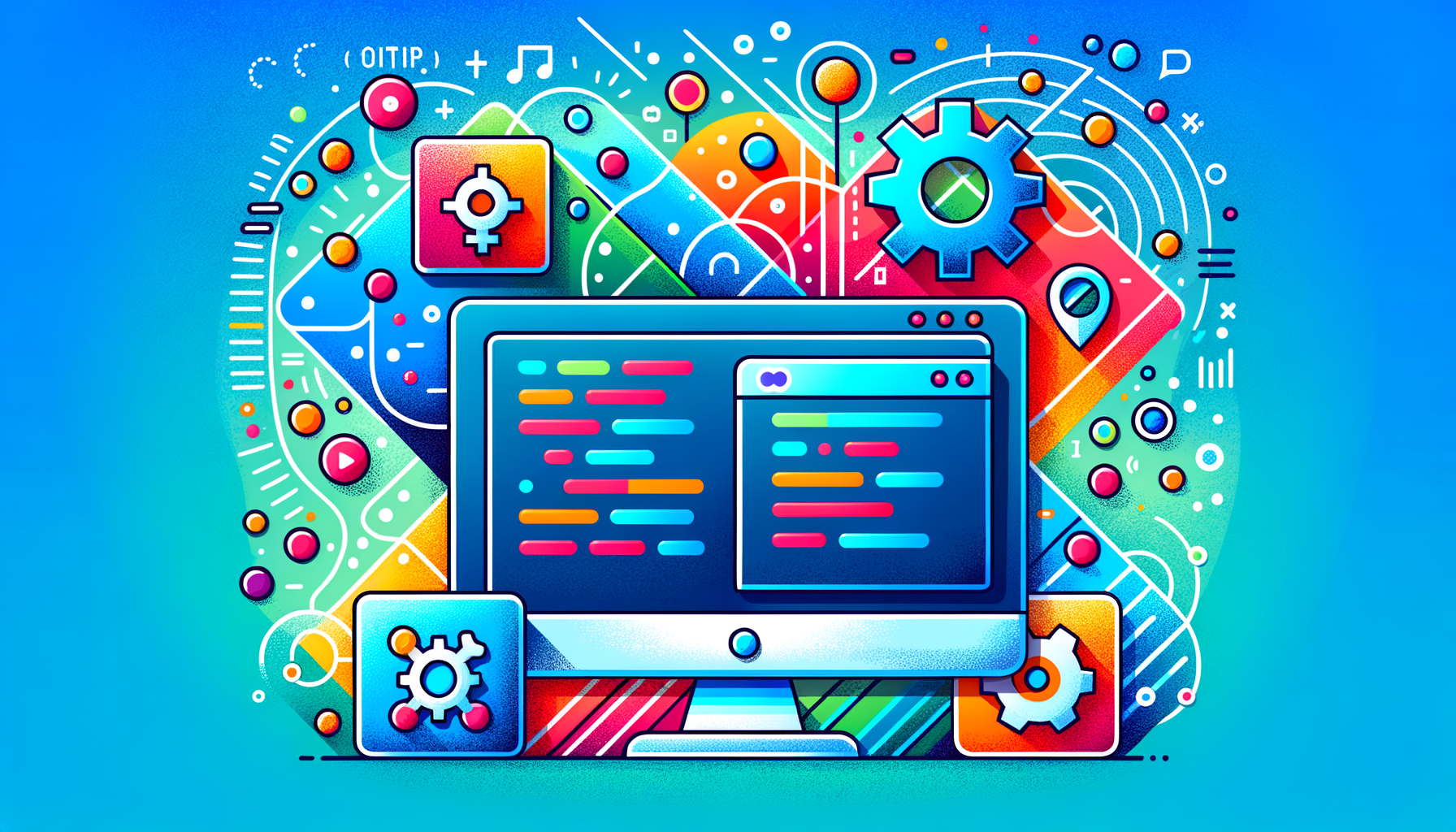
Customizing Your WordPress Site with Widgets
Widgets are a powerful tool in WordPress, allowing you to customize and enhance the user interface of your website. Whether you’re looking to add a simple text box, a complex form, or a dynamic feed, creating custom WordPress widgets can help you achieve your goals. Here’s a comprehensive guide on how to develop custom WordPress widgets, complete with step-by-step instructions and real-world examples.
Understanding the Basics of Widget Development
Before diving into the code, it’s essential to understand the basics of widget development in WordPress. Widgets are PHP objects that extend the WP_Widget
class, which is located in the wp-includes/class-wp-widget.php
file of your WordPress installation.
To start, you’ll need a few tools:
- A text editor for editing your code
- Access to your WordPress admin dashboard
- A Secure File Transfer Protocol (SFTP) client for accessing your site’s files directly
- Familiarity with the basics of classes and objects in PHP5.
Step 1: Setting Up Your Widget
The first step in creating a custom widget is to set up the base code. You can either create a new plugin to house your widget or paste the code directly into your theme’s functions.php
file. However, creating a plugin is generally recommended as it allows you to use the widget with any theme.
Here’s how you can set up your widget plugin:
<?php
/*
Plugin Name: My Custom Widget
Plugin URI: https://www.example.com/my-custom-widget
Description: A custom widget for WordPress.
Version: 1.0
Author: Your Name
Author URI: https://www.example.com
License: GPL2
*/
Create a new folder in your wp-content/plugins/
directory and add this header code to the main PHP file of your plugin.
Step 2: Extending the WP_Widget Class
To create your custom widget, you need to extend the WP_Widget
class. Here’s an example of how you can define your widget class:
<?php
class My_Custom_Widget extends WP_Widget {
public function __construct() {
parent::__construct(
'my_custom_widget',
__('My Custom Widget', 'text_domain'),
array('description' => __('A custom widget for your site.', 'text_domain'))
);
}
public function widget($args, $instance) {
// Output the widget content here
echo $args['before_widget'];
if (!empty($instance['title'])) {
echo $args['before_title'] . apply_filters('widget_title', $instance['title']) . $args['after_title'];
}
// Your widget content goes here
echo $args['after_widget'];
}
public function form($instance) {
// Create the form fields for the widget settings
$title = !empty($instance['title']) ? $instance['title'] : __('New title', 'text_domain');
?>
<p>
<label for="<?php echo $this->get_field_id('title'); ?>"><?php _e('Title:'); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id('title'); ?>" name="<?php echo $this->get_field_name('title'); ?>" type="text" value="<?php echo esc_attr($title); ?>">
</p>
<?php
}
public function update($new_instance, $old_instance) {
// Update the widget settings
$instance = array();
$instance['title'] = (!empty($new_instance['title'])) ? strip_tags($new_instance['title']) : '';
return $instance;
}
}
This code defines the basic structure of your widget, including the constructor, the widget output, the form fields, and the update function.
Step 3: Registering Your Widget
To make your widget available in the WordPress admin, you need to register it using the widgets_init
hook:
<?php
add_action('widgets_init', function() {
register_widget('My_Custom_Widget');
});
This code registers your widget so that it appears in the list of available widgets in your WordPress dashboard.
Step 4: Customizing and Testing Your Widget
Now that your widget is set up and registered, you can customize it further to meet your specific needs. Here are a few key areas to focus on:
Using WordPress Hooks
WordPress hooks allow you to ‘hook in’ to WordPress’ core code and enact specific functions at certain points. For example, you can use the widget
method to define the output of your widget, and the form
method to create settings fields in the admin area.
Adding Custom Functionality
You can add custom functionality to your widget by modifying the widget
method. For instance, you might want to display a custom title, fetch data from an external API, or include dynamic content.
public function widget($args, $instance) {
// Fetch data from an external API
$data = json_decode(file_get_contents('https://api.example.com/data'), true);
echo $args['before_widget'];
if (!empty($instance['title'])) {
echo $args['before_title'] . apply_filters('widget_title', $instance['title']) . $args['after_title'];
}
// Display the fetched data
echo '<ul>';
foreach ($data as $item) {
echo '<li>' . $item['name'] . '</li>';
}
echo '</ul>';
echo $args['after_widget'];
}
Optimizing Performance
To ensure your widget performs well, especially if it involves complex operations or external requests, consider optimizing your code. For example, you might use caching to reduce the load on your server.
public function widget($args, $instance) {
// Use caching to reduce server load
$cache_key = 'my_widget_data';
$data = get_transient($cache_key);
if ($data === false) {
$data = json_decode(file_get_contents('https://api.example.com/data'), true);
set_transient($cache_key, $data, 3600); // Cache for 1 hour
}
echo $args['before_widget'];
if (!empty($instance['title'])) {
echo $args['before_title'] . apply_filters('widget_title', $instance['title']) . $args['after_title'];
}
// Display the cached data
echo '<ul>';
foreach ($data as $item) {
echo '<li>' . $item['name'] . '</li>';
}
echo '</ul>';
echo $args['after_widget'];
}
Real-World Examples and Case Studies
Example 1: Displaying Site Information
Here’s an example of a widget that displays the site title and tagline:
class Site_Info_Widget extends WP_Widget {
public function __construct() {
parent::__construct(
'site_info_widget',
__('Site Info Widget', 'text_domain'),
array('description' => __('Displays site title and tagline.', 'text_domain'))
);
}
public function widget($args, $instance) {
echo $args['before_widget'];
echo '<h4>' . get_bloginfo('name') . '</h4>';
echo '<p>' . get_bloginfo('description') . '</p>';
echo $args['after_widget'];
}
}
Example 2: Integrating with Third-Party Services
You can also integrate your widget with third-party services. For instance, you might create a widget that displays social media feeds using APIs from platforms like Twitter or Instagram.
class Social_Media_Feed_Widget extends WP_Widget {
public function __construct() {
parent::__construct(
'social_media_feed_widget',
__('Social Media Feed Widget', 'text_domain'),
array('description' => __('Displays social media feed.', 'text_domain'))
);
}
public function widget($args, $instance) {
// Fetch data from social media API
$feed = json_decode(file_get_contents('https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name=example&count=5'), true);
echo $args['before_widget'];
echo '<ul>';
foreach ($feed as $tweet) {
echo '<li>' . $tweet['text'] . '</li>';
}
echo '</ul>';
echo $args['after_widget'];
}
}
Best Practices and Tools
Using Secure Hosting
To ensure your website and widgets perform optimally, consider using a secure and reliable hosting service like Kinsta. Kinsta offers WordPress-optimized hosting plans that include automatic backups, staging environments, and 24/7 support.
Leveraging Code Snippet Plugins
For managing and inserting code snippets on your site without risking errors, you can use plugins like WPCode. This plugin helps you manage custom code snippets efficiently.
Testing in Staging Environments
Always test your custom widgets in a staging environment before deploying them to your live site. This ensures that any errors or glitches are caught and fixed before they affect your users.
Conclusion and Next Steps
Creating custom WordPress widgets is a powerful way to enhance the functionality and user interface of your website. By following these steps and best practices, you can develop widgets that meet your specific needs and improve the overall user experience.
If you need further assistance or have complex requirements for your widgets, consider reaching out to a professional WordPress development agency like Belov Digital Agency for customized solutions. You can also contact us for more information on how we can help you with your WordPress projects.
Remember, the key to successful widget development is careful planning, thorough testing, and adherence to best practices. Happy coding