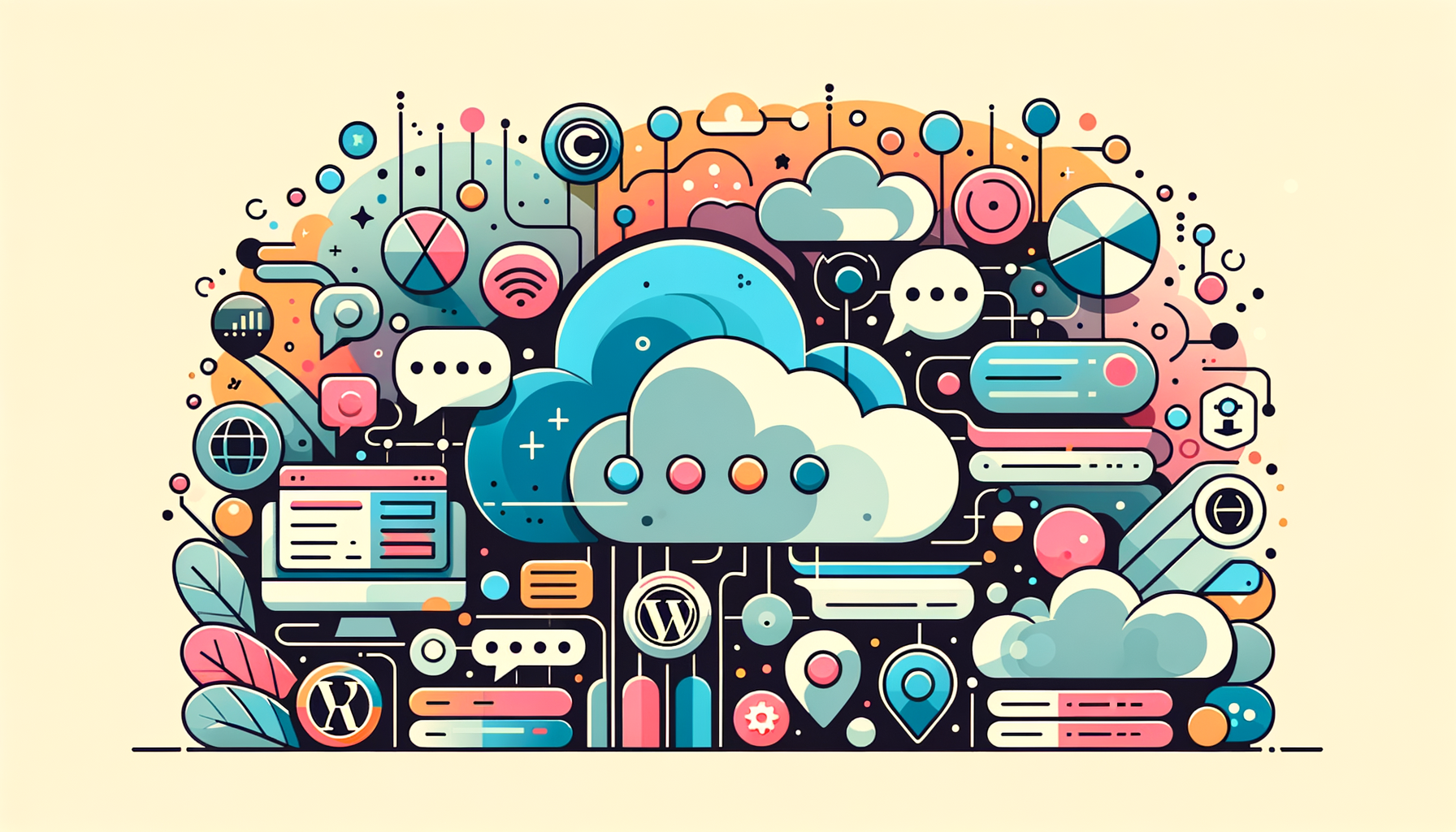
Enhancing WordPress with Real-Time Communication: A Guide to Implementing WebSockets
In the modern web development landscape, providing real-time updates and interactive features is crucial for enhancing user engagement and experience. WebSockets, a protocol that enables full-duplex communication between a client and a server, are ideal for achieving this. Here’s a comprehensive guide on implementing WebSockets in WordPress to add real-time communication capabilities to your website.
Understanding WebSockets
WebSockets facilitate real-time, two-way communication between a server and clients, making them perfect for live features such as notifications, chat applications, and real-time updates. Unlike traditional HTTP, WebSockets maintain an open connection, allowing for continuous data exchange without the need for frequent or long polling.
Choosing the Right WebSocket Server
To implement WebSockets, you need to choose a suitable WebSocket server. Node.js, along with libraries like Socket.io, is a popular choice due to its ease of use and robust features.
Setting Up a WebSocket Server with Node.js
- Install Node.js and Socket.io:
npm install socket.io
- Create a Basic WebSocket Server:
const io = require('socket.io')(3000); io.on('connection', socket => { console.log('New client connected'); socket.on('message', msg => { console.log('Message received:', msg); io.emit('message', msg); }); });
- Client-Side Integration:
const socket = io('http://yourdomain.com:3000'); socket.on('message', msg => { console.log('New message:', msg); });
Embed this script in your WordPress theme or plugin to enable real-time communication.
Connecting WordPress to the WebSocket Server
To connect your WordPress site to the WebSocket server, you need to integrate the client-side JavaScript into your WordPress theme or plugin.
Using JavaScript in WordPress
You can add the JavaScript code to your WordPress site by including it in the footer.php
or header.php
file of your theme, or by using a plugin like Custom JavaScript to manage your scripts.
This script establishes a connection to the WebSocket server and listens for incoming messages.
Configuring Web Servers for WebSocket Support
WebSockets require specific configurations on your web server to function correctly.
Apache HTTP Server
To enable WebSocket support on Apache, ensure that the mod_proxy
and mod_proxy_wstunnel
modules are enabled. Add a proxy rule to forward WebSocket connections to the appropriate backend server:
ProxyPass /socket/ ws://localhost:8080/
ProxyPassReverse /socket/ ws://localhost:8080/
Reload or restart Apache to apply the configuration changes.
Nginx
For Nginx, add a location block to your configuration file to proxy WebSocket connections:
location /socket/ {
proxy_pass http://localhost:8080;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection "Upgrade";
}
Reload or restart Nginx to apply the changes.
Microsoft IIS
Ensure that the WebSocket Protocol is installed as a server role in the Windows Server Manager. Enable the WebSocket feature in the IIS Manager and configure any necessary settings such as request timeout or buffer size.
Handling Connection Disruptions
WebSocket connections are long-lived but can be disrupted by events like deploys, autoscaling, or network issues. Your application code should be designed to reestablish WebSocket connections automatically when they are disrupted.
socket.on('disconnect', () => {
setTimeout(() => {
socket.connect();
}, 5000); // Reconnect after 5 seconds
});
This ensures that your application remains connected and functional even in the face of disruptions.
Security and Best Practices
WebSockets utilize the same security mechanisms as HTTP, such as SSL/TLS encryption, ensuring the confidentiality and integrity of data exchanged between clients and servers. Here are some best practices to keep in mind:
- Use SSL/TLS: Ensure that your WebSocket connections are encrypted using SSL/TLS to protect data.
- Validate Data: Always validate data received from clients to prevent security vulnerabilities.
- Use Secure Paths: Use secure paths for WebSocket connections, such as those starting with
/_ws/
or/socket.io/
.
Real-World Examples and Case Studies
Live Chat Systems
Implementing WebSockets for live chat systems allows for real-time communication between users. For example, you can use Socket.io to create a chat application where messages are sent and received in real-time.
socket.on('message', msg => {
const chatLog = document.getElementById('chat-log');
const messageElement = document.createElement('div');
messageElement.textContent = msg;
chatLog.appendChild(messageElement);
});
This code snippet updates the chat log in real-time as messages are received from the server.
Real-Time Notifications
WebSockets can be used to push real-time notifications to users. For instance, you can notify users of new comments on a blog post or updates to a collaborative document.
socket.on('notification', notification => {
const notificationElement = document.createElement('div');
notificationElement.textContent = notification.message;
document.body.appendChild(notificationElement);
});
This code snippet displays a notification to the user as soon as it is received from the server.
Conclusion and Next Steps
Implementing WebSockets in WordPress can significantly enhance the interactivity of your site by enabling real-time communication. By following the steps outlined above and adhering to best practices, you can create robust and engaging real-time applications.
If you need further assistance or have complex requirements, consider reaching out to a professional WordPress development agency like Belov Digital Agency for customized solutions. For more detailed guides and tutorials, you can also visit our blog or contact us for a consultation.
Additionally, if you are looking for reliable hosting solutions that support WebSocket connections, consider using services like Kinsta, which offers optimized environments for real-time applications.
By leveraging WebSockets, you can take your WordPress site to the next level, providing users with a more interactive and engaging experience.