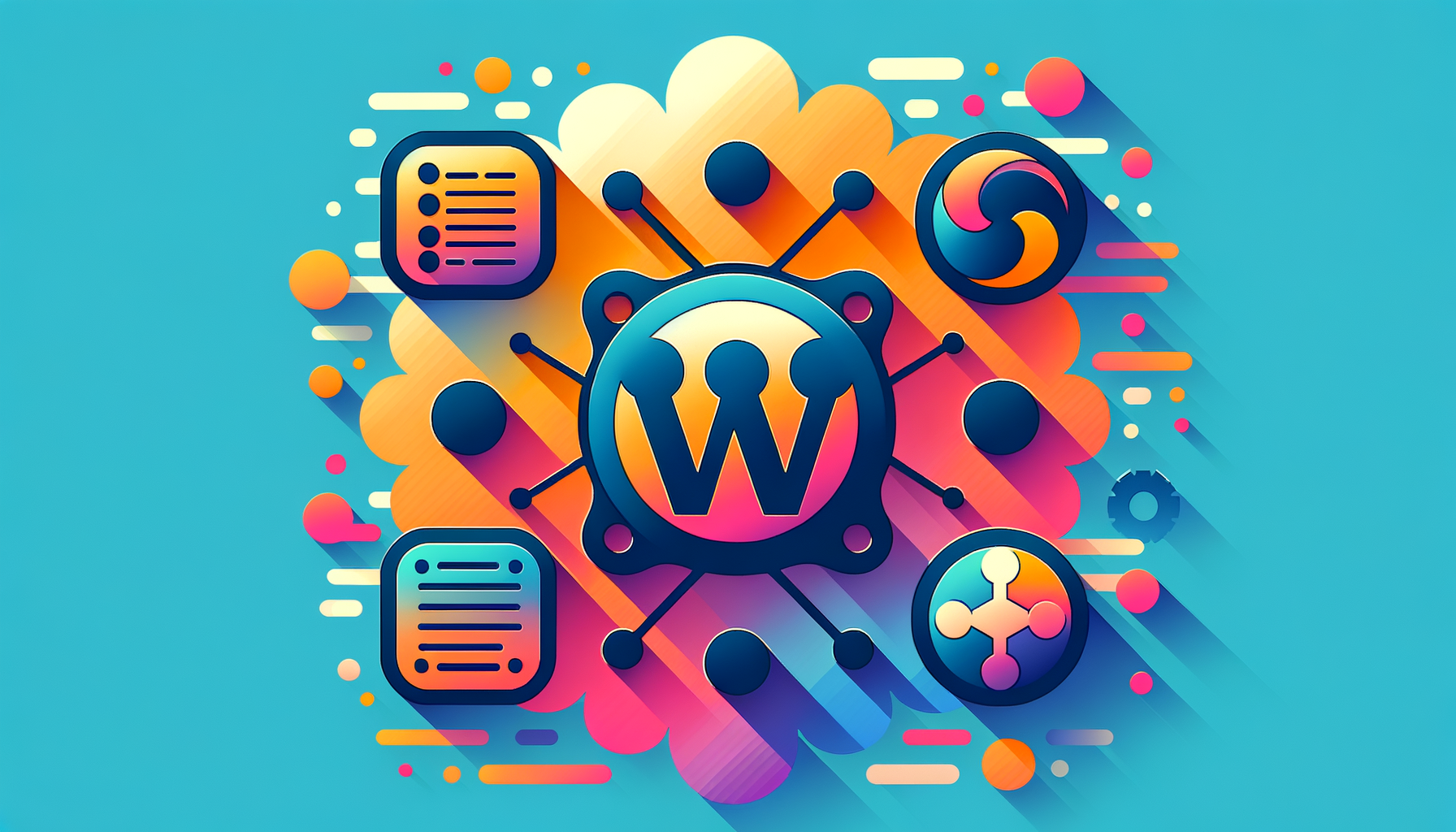
Enhancing User Engagement with Real-Time Communication in WordPress
In the ever-evolving landscape of web development, providing real-time updates and interactive features has become a cornerstone for enhancing user engagement and experience. One of the most effective ways to achieve this is by implementing WebSockets in your WordPress projects. Here’s a comprehensive guide on how to integrate WebSockets into your WordPress site, along with real-world examples and best practices.
Understanding WebSockets
WebSockets are a protocol that facilitates full-duplex communication between a client and a server, allowing for real-time, two-way communication. Unlike traditional HTTP, which relies on request-response cycles, WebSockets maintain an open connection, enabling continuous data exchange without the need for frequent or long polling.
Setting Up a WebSocket Server
To implement WebSockets in your WordPress project, you first need to set up a WebSocket server. Here’s how you can do it using Node.js and Socket.io:
Installing Node.js and Socket.io
To get started, you need to install Node.js and Socket.io. You can do this by running the following command in your terminal:
npm install socket.io
Creating a Basic WebSocket Server
Here’s an example of how to create a basic WebSocket server using Socket.io:
const io = require('socket.io')(3000);
io.on('connection', socket => {
console.log('New client connected');
socket.on('message', msg => {
console.log('Message received:', msg);
io.emit('message', msg);
});
});
This script sets up a WebSocket server on port 3000 and logs messages received from clients, broadcasting them back to all connected clients.
Client-Side Integration
To connect your WordPress site to the WebSocket server, you need to integrate the client-side JavaScript into your WordPress theme or plugin. Here’s how you can do it:
const socket = io('http://yourdomain.com:3000');
socket.on('message', msg => {
console.log('New message:', msg);
});
You can add this script to your WordPress site by including it in the footer.php
or header.php
file of your theme, or by using a plugin like Custom JavaScript to manage your scripts.
Configuring Web Servers for WebSocket Support
WebSockets require specific configurations on your web server to function correctly.
Apache HTTP Server
For Apache, ensure that the mod_proxy
and mod_proxy_wstunnel
modules are enabled. Add a proxy rule to forward WebSocket connections to the appropriate backend server:
ProxyPass /socket/ ws://localhost:8080/
ProxyPassReverse /socket/ ws://localhost:8080/
Reload or restart Apache to apply the configuration changes.
Nginx
For Nginx, add a location block to your configuration file to proxy WebSocket connections:
location /socket/ {
proxy_pass http://localhost:8080;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection "Upgrade";
}
Reload or restart Nginx to apply the changes.
Real-World Examples and Case Studies
Live Chat Systems
Implementing WebSockets for live chat systems allows for real-time communication between users. For example, you can use Socket.io to create a chat application where messages are sent and received in real-time. Here’s a snippet of how you can update the chat log in real-time:
socket.on('message', msg => {
const chatLog = document.getElementById('chat-log');
const messageElement = document.createElement('div');
messageElement.textContent = msg;
chatLog.appendChild(messageElement);
});
This code updates the chat log in real-time as messages are received from the server.
Real-Time Notifications
WebSockets can also be used to push real-time notifications to users. For instance, you can notify users of new comments on a blog post or updates to a collaborative document:
socket.on('notification', notification => {
const notificationElement = document.createElement('div');
notificationElement.textContent = notification.message;
document.body.appendChild(notificationElement);
});
This code snippet displays a notification to the user as soon as it is received from the server.
Real-Time Private Messaging Systems
For more advanced use cases, such as real-time private messaging systems, you can integrate plugins like RumbleTalk into your WordPress site. RumbleTalk offers features like real-time messaging, multimedia sharing, and private chat rooms, which can significantly enhance user engagement and community interaction within your closed community.
Hosting Considerations
When choosing a hosting service for your WordPress site with WebSocket capabilities, it’s crucial to select a provider that supports WebSocket connections. Services like Kinsta offer optimized environments for real-time applications, ensuring that your WebSocket implementation runs smoothly and efficiently.
Security and Best Practices
Implementing WebSockets also requires careful consideration of security. Here are some best practices to keep in mind:
- Authentication and Authorization: Ensure that only authenticated users can connect to your WebSocket server.
- Data Encryption: Use SSL/TLS to encrypt data transmitted over WebSockets.
- Rate Limiting: Implement rate limiting to prevent abuse and denial-of-service attacks.
For more detailed guides on security and best practices, you can refer to resources like OWASP WebSocket Security Guide.
Conclusion and Next Steps
Implementing WebSockets in your WordPress project can significantly enhance the interactivity of your site by enabling real-time communication. By following the steps outlined above and adhering to best practices, you can create robust and engaging real-time applications.
If you need further assistance or have complex requirements, consider reaching out to a professional WordPress development agency like Belov Digital Agency for customized solutions. For more detailed guides and tutorials, you can also visit our blog or contact us for a consultation.
By leveraging WebSockets, you can take your WordPress site to the next level, providing users with a more interactive and engaging experience. Whether you’re building a live chat system, real-time notifications, or dynamic content updates, WebSockets are the key to unlocking real-time communication in your web applications.