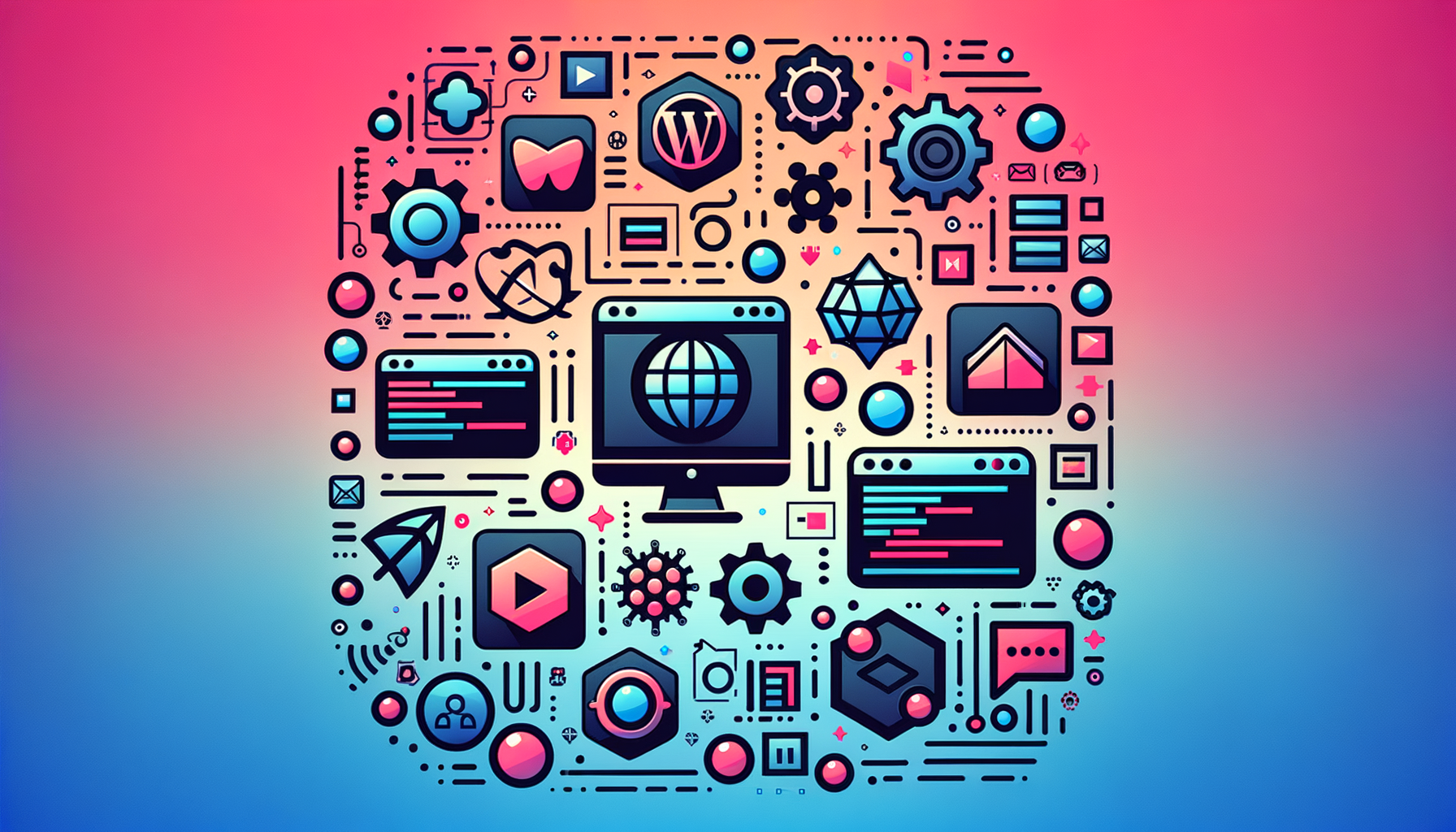
Leveraging Node.js for Advanced WordPress Development
In the ever-evolving landscape of web development, the integration of Node.js with WordPress has opened up a plethora of possibilities for creating dynamic, interactive, and highly scalable web applications. This guide will delve into the details of how to harness the power of Node.js in your WordPress development projects, enhancing both the front-end and back-end capabilities of your site.
Why Merge Node.js and WordPress?
Node.js, known for its speed, scalability, and real-time capabilities, is an ideal complement to WordPress, which offers a robust and user-friendly content management system (CMS). By combining these two technologies, you can achieve a dynamic, interactive front-end powered by Node.js and a manageable back-end managed by WordPress.
- Real-Time Capabilities: Node.js is renowned for its ability to handle real-time data, making it perfect for features like live updates, chat applications, and dynamic content.
- Scalability: Node.js can handle high traffic and load efficiently, which is particularly beneficial for sites experiencing heavy user engagement.
- Customization: With Node.js, you can customize your WordPress site to a greater extent, including user-specific content and site administration tasks.
Setting Up Your Environment
Before diving into the integration, ensure you have the necessary tools and environments set up.
Installing Node.js and npm
To start, you need to install Node.js and npm (Node Package Manager) on your computer. Here’s a step-by-step guide for different operating systems:
- Windows: Download Node.js from the official website, follow the setup wizard, and ensure it is added to your system path. You can verify the installation by running
node -v
andnpm -v
in your terminal. - macOS and Linux: Use the terminal to install Node.js. For macOS, you can use Homebrew, while for Linux, you can use the package manager of your distribution.
Setting Up Your WordPress Site
Ensure you have a running WordPress site. If you’re developing locally, tools like wp-env
or wp-now
can help you set up a local WordPress environment efficiently.
Creating a Node.js Application
To integrate Node.js with your WordPress site, you need to create a Node.js application that will handle the dynamic functionalities.
Basic Node.js Server Setup
Here’s an example of setting up a basic Node.js server using Express.js:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World');
});
app.listen(port, () => {
console.log(`Node.js server is running on port ${port}`);
});
This code sets up an Express server that listens on port 3000 and responds with “Hello World” to GET requests to the root URL.
Connecting Node.js to WordPress
The key to integrating Node.js with WordPress is the WordPress REST API. This API allows your Node.js application to interact with your WordPress site programmatically.
Using the WordPress REST API
To fetch posts from your WordPress site, you can use the Axios library to make HTTP requests to the WordPress REST API:
const axios = require('axios');
const wordpressApiUrl = 'https://your-wordpress-site.com/wp-json/wp/v2/posts';
axios.get(wordpressApiUrl)
.then((response) => {
const posts = response.data;
console.log(posts);
})
.catch((error) => {
console.error('Error fetching posts:', error);
});
This code fetches the list of posts from your WordPress site and logs them to the console.
Managing WordPress Posts with Node.js
You can create specific endpoints in your Node.js application to manage WordPress posts.
Creating a New Post
To create a new post in WordPress, you can set up an endpoint in your Node.js server that makes a POST request to the /posts
endpoint of the WordPress REST API:
app.post("/add-post", async (req, res) => {
try {
const postID = req.body.id;
const resp = await axios.post(`https://yourdomain.com/wp-json/wp/v2/posts/${postID}`, req.body);
if (resp.status !== 200) throw "Something went wrong";
} catch (err) {
console.log(err);
}
});
This code creates an endpoint /add-post
that extracts the post details from the request body and sends a POST request to your WordPress site to create a new post.
Customizing Sites for Users
You can also use Node.js to customize your WordPress site based on user preferences.
Storing User Data
You can store user data in cookies or sessions and use this data to customize the content displayed to each user:
app.post("/sign-up", async (req, res) => {
// Sign up user
res.cookie("cookie_id", 123456);
res.cookie("lang", req.body.language);
res.status(200).json("Logged in.");
});
This code sets cookies for the user’s ID and preferred language, which can then be used to customize the content.
Incorporating Real-Time Functionalities
One of the major benefits of using Node.js is its ability to handle real-time data. You can integrate features like live notifications, chat applications, or dynamic content updates using WebSockets and libraries like Socket.io.
const io = require('socket.io')(app);
io.on('connection', (socket) => {
console.log('a user connected');
socket.on('disconnect', () => {
console.log('user disconnected');
});
});
This code sets up a basic WebSocket connection using Socket.io, enabling real-time communication between the server and clients.
Deployment and Scaling
Once you have developed your integrated Node.js and WordPress application, it’s crucial to deploy it on a scalable hosting platform.
Hosting Options
You can use hosting platforms like Kinsta, Heroku, AWS, or DigitalOcean to ensure your application scales efficiently. Kinsta, in particular, offers robust application hosting that can handle high traffic and load.
Conclusion and Next Steps
Integrating Node.js with WordPress opens up a world of possibilities for dynamic and interactive web applications. By following the steps outlined above, you can leverage the strengths of both technologies to enhance your site’s performance and user experience.
- Seek Professional Help: If the integration process seems challenging, consider hiring a professional Node.js developer from Belov Digital Agency to ensure a seamless integration and optimal performance.
- Explore Further: Don’t hesitate to explore more advanced features such as advanced searching, theme manipulation, and post revisions using the WordPress REST API Handbook.
- Optimize Performance: Always ensure your server is optimized for performance. Tools like caching plugins and CDNs can significantly improve your site’s responsiveness.
By combining the flexibility of Node.js with the robustness of WordPress, you can create web applications that are not only dynamic and interactive but also highly scalable and performant. For more detailed guides and tutorials, you can visit our blog or contact us for professional assistance.