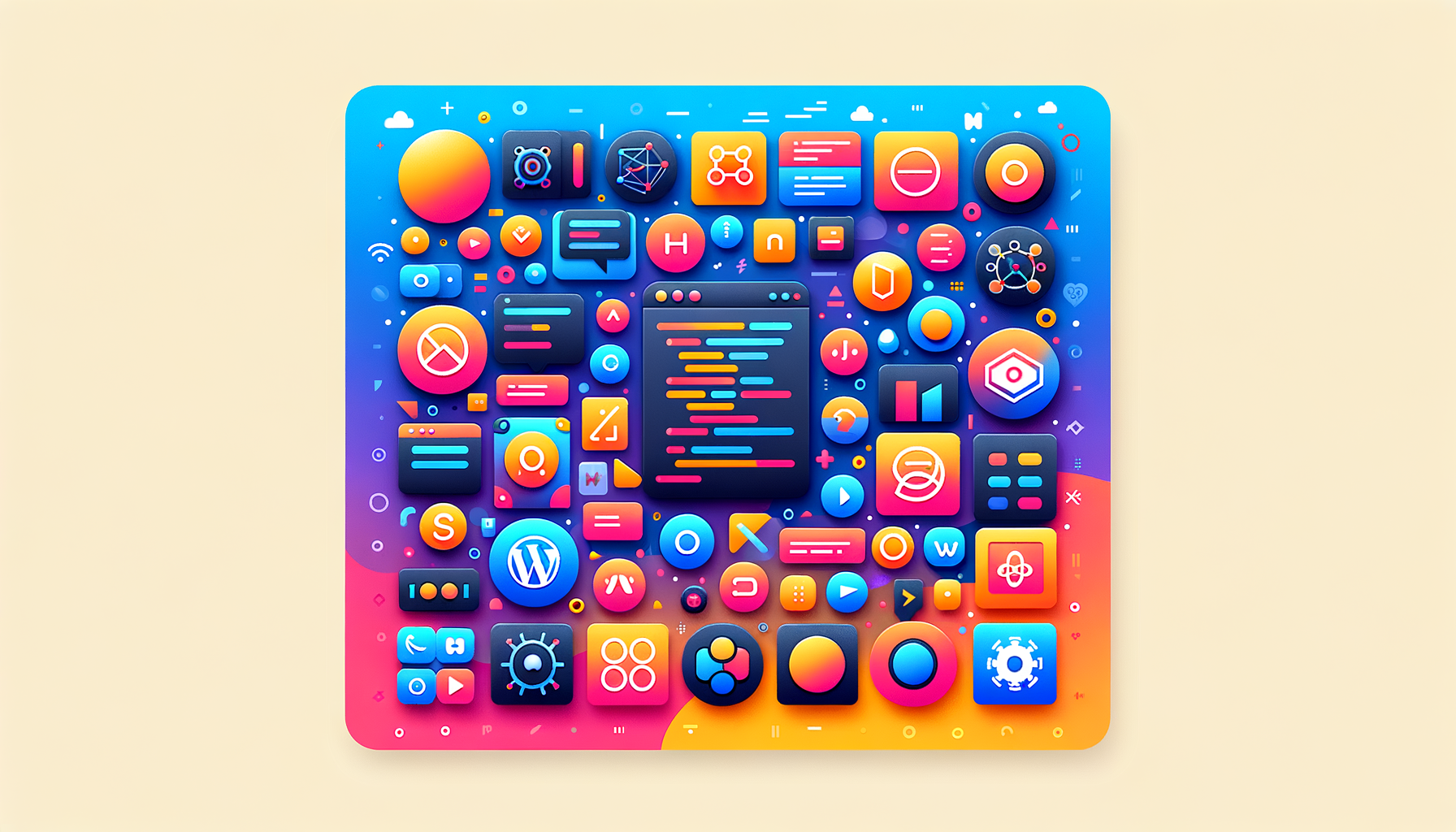
Leveraging React for Advanced WordPress Development
In the ever-evolving landscape of web development, integrating WordPress with modern JavaScript frameworks like React has become a game-changer. This approach, often referred to as “headless WordPress,” allows developers to harness the powerful content management capabilities of WordPress while leveraging the dynamic and interactive user interface (UI) components of React. Here’s a comprehensive guide on how to create headless WordPress themes using React.
Understanding React and WordPress REST API
Before diving into the integration process, it’s essential to understand the basics of React and the WordPress REST API.
React, developed by Facebook, is a user interface library that uses a component-based approach to build scalable and cross-platform applications. It is particularly beneficial for its simplicity, reusability of components, and the use of JSX, which integrates HTML and JavaScript.
The WordPress REST API (WP REST API) is a set of protocols that enable developers to interact with WordPress data from the frontend. It allows you to access, create, update, and delete WordPress content using JSON (JavaScript Object Notation) format. To use the WP REST API, ensure that your WordPress permalinks are enabled and set to something other than “Plain” by navigating to Settings > Permalinks in your WordPress admin panel.
Setting Up Your Development Environment
To start developing a headless WordPress theme with React, you need a few tools and dependencies:
- Node.js and npm: These are essential for managing JavaScript packages and running your React application.
- Text Editor: Choose a text editor like Visual Studio Code or Sublime Text.
- Git: Optional but recommended for version control.
- Create React App (CRA): A popular tool for setting up a React environment quickly. You can create a new project using the command
npx create-react-app wp-react-demo
.
For a more integrated development experience, you can use tools like DevKinsta from Kinsta, which provides a user-friendly local development environment for WordPress and React projects.
Integrating React with WordPress
Using WP REST API
To integrate React with WordPress, you need to set up the WP REST API. Here’s how you can do it:
- Ensure your WordPress site has the REST API enabled by checking your permalink settings.
- Use the
@wordpress/scripts
package to simplify the configuration and build process. This package provides reusable scripts tailored for WordPress development.
Here is an example of how you can enqueue your React script in the functions.php
file of your WordPress theme:
<?php
function my_react_theme_scripts() {
wp_enqueue_script('my-react-theme-app', get_template_directory_uri() . '/build/index.js', array('wp-element'), '1.0.0', true);
wp_enqueue_style('my-react-theme-style', get_stylesheet_uri());
}
add_action('wp_enqueue_scripts', 'my_react_theme_scripts');
?>
Creating React Components
Inside your theme directory, create a src
folder for your React source files. Here, you can create files like index.js
and App.js
.
// index.js
import { render } from '@wordpress/element';
import App from './App';
render(<App />, document.getElementById('app'));
// App.js
import { Component } from '@wordpress/element';
export default class App extends Component {
render() {
return (
<div>
<h1>Hello, WordPress and React!</h1>
{/* Your React components will go here */}
</div>
);
}
}
This setup mounts your React App
component to the DOM, allowing you to start building your interactive UI.
Handling WordPress Data in React
To fetch and display WordPress data in your React application, you can use the WP REST API. Here’s an example of how to fetch posts:
// Posts.js
import React, { useState, useEffect } from 'react';
const Posts = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
fetch('https://yourwordpresssite.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(data => setPosts(data));
}, []);
return (
<div>
{posts.map(post => (
<div key={post.id}>
<h2>{post.title.rendered}</h2>
<p>{post.excerpt.rendered}</p>
</div>
))}
</div>
);
};
export default Posts;
This code fetches posts from the WP REST API and displays them in your React component.
Creating Custom Gutenberg Blocks with React
Another powerful way to extend WordPress functionality is by creating custom Gutenberg blocks using React. Here’s a step-by-step guide:
- Create a new WordPress plugin or theme folder.
- Initialize a new npm project inside the folder.
- Install the
@wordpress/scripts
package. - Create a PHP file to register your block with WordPress.
- Set up your block’s React component and compile it using
wp-scripts
.
Here’s an example of how to register a block in WordPress:
// my-custom-block.php
function register_my_custom_block() {
wp_enqueue_script(
'my-custom-block',
plugins_url('build/index.js', __FILE__),
array('wp-element', 'wp-blocks', 'wp-editor'),
'1.0.0',
true
);
register_block_type('my-plugin/my-custom-block', array(
'editor_script' => 'my-custom-block',
));
}
add_action('init', 'register_my_custom_block');
Using ReactPress for Seamless Integration
For a more streamlined experience, you can use the ReactPress plugin, which integrates your local development server into your WordPress theme, providing instant feedback and easy deployment options.
ReactPress offers features like fast refresh during app development, WordPress integration during development, and easy deployment to your live site. It also supports client-side routing and TypeScript.
Real-World Examples and Case Studies
E-commerce Store with WordPress and React
Imagine building an e-commerce store where you use WordPress as the backend to manage products and React to create a dynamic and interactive frontend. You can use the WP REST API to fetch product data and display it in your React application. This setup allows for a seamless user experience and efficient management of your online store.
Interactive Blog with Custom Gutenberg Blocks
You can create a highly interactive blog by developing custom Gutenberg blocks using React. These blocks can include features like dynamic content loading, interactive elements, and real-time updates, enhancing the user experience significantly.
Conclusion and Next Steps
Integrating React with WordPress opens up a world of possibilities for web development. By leveraging the strengths of both platforms, you can create modern, interactive, and performant websites.
If you need assistance with your WordPress and React projects, consider reaching out to experts at Belov Digital Agency, who specialize in WordPress development and can help you from plugin creation to custom theme development.
For hosting your WordPress site, consider using Kinsta, which offers robust and scalable hosting solutions tailored for WordPress and React applications.
By following the steps outlined above and exploring tools like Create React App and ReactPress, you can create powerful headless WordPress themes that elevate your web development projects.
Additional Resources
- WordPress REST API Documentation: For detailed information on using the WP REST API, visit the official WordPress REST API documentation.
- React Official Documentation: For a comprehensive guide to React, check out the official React documentation.
- Kinsta Tutorials: For more tutorials on integrating React with WordPress, visit Kinsta’s blog.
By combining the flexibility of WordPress with the powerful UI capabilities of React, you can create web applications that are both functional and visually appealing. Start your journey today and explore the endless possibilities of headless WordPress development with React.