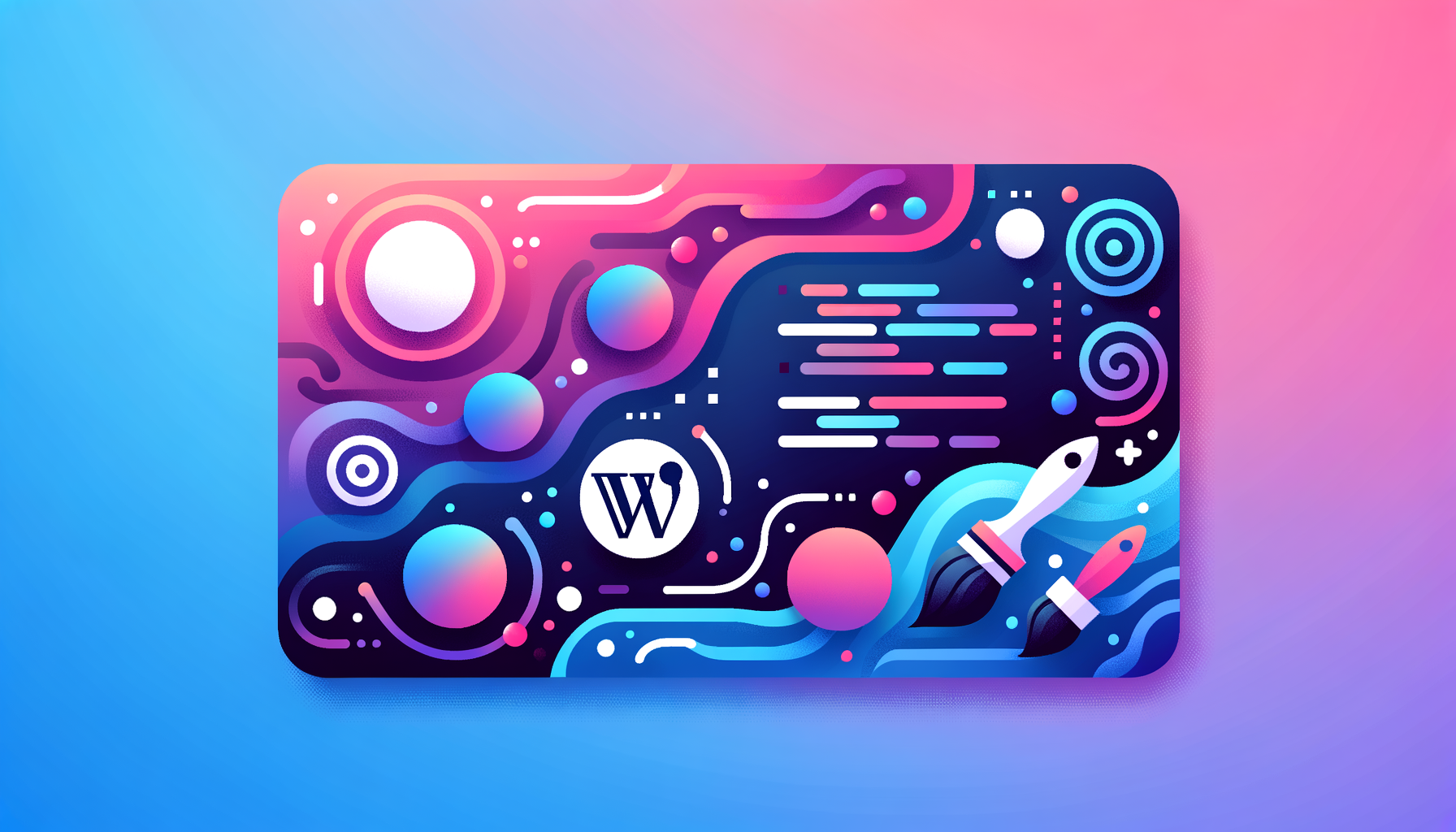
Streamlining Your WordPress Development with Sass
When it comes to developing WordPress themes, managing CSS can quickly become a daunting task. This is where Sass, a powerful CSS preprocessor, comes into play. In this article, we will delve into the world of Sass, exploring how it can revolutionize your WordPress development process.
What is Sass and Why Use It?
Sass, or Syntactically Awesome Stylesheets, is a stylesheet language that compiles to CSS. It offers a range of features that make CSS development more efficient, such as variables, nested rules, mixins, and functions. These features help keep large stylesheets well-organized and make it easier to share design elements across projects.
For example, with Sass, you can define a variable for a color and use it throughout your stylesheet, making it easier to maintain consistency and make changes.
$primary-color: #333;
body {
color: $primary-color;
}
Setting Up Sass for WordPress
To use Sass in your WordPress theme, you need to set up a few things.
Prerequisites
Before you start, ensure you have a working knowledge of setting up a WordPress environment. You can find detailed guides on setting up a local WordPress install on websites like WPBeginner.
Installing Sass and Compass
Sass has a Ruby dependency, so you need to install the Ruby gem. On Macs, Ruby is often pre-installed, but on Windows, you may need to install it separately. You can also use frameworks like Compass or Bourbon to enhance your Sass experience.
Here’s how you can install Sass and Compass:
gem install sass
gem install compass
Folder Structure and Files
Create a sass
folder within your WordPress theme directory. Inside this folder, create a style.scss
file, which will be your main Sass file. This file will be compiled into style.css
, which WordPress can use.
wp-content/themes/your-theme/
├── sass/
│ ├── style.scss
│ ├── _partials.scss
├── style.css
In your style.scss
file, you can import partials and other Sass files:
@use 'partials';
Compiling Sass to CSS
WordPress cannot use Sass files directly, so you need to compile them to CSS. You can use tools like Koala, CodeKit, or command-line tools like sass
or task runners like Gulp.
For example, using Gulp, you can set up a gulpfile.js
to automate the compilation process:
const gulp = require('gulp');
const sass = require('gulp-sass');
gulp.task('sass', function() {
return gulp.src('./sass/style.scss')
.pipe(sass().on('error', sass.logError))
.pipe(gulp.dest('./'));
});
You can also use a simple command-line watcher:
sass --watch sass/style.scss:style.css
Key Features of Sass
Variables
Variables in Sass allow you to store values that you can reuse throughout your stylesheet. This is particularly useful for colors, font stacks, and other CSS values that you might need to change frequently.
$font-stack: Helvetica, sans-serif;
$primary-color: #333;
body {
font: 100% $font-stack;
color: $primary-color;
}
Nesting
Sass allows you to nest your CSS selectors, which makes your code more readable and easier to manage.
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
li {
display: inline-block;
a {
display: block;
padding: 6px 12px;
text-decoration: none;
}
}
}
}
Mixins
Mixins are reusable blocks of CSS that you can include in your selectors. They are particularly useful for vendor prefixes and other repetitive styles.
@mixin flexbox {
display: flex;
justify-content: center;
align-items: center;
}
.button {
@include flexbox;
background: #fff;
&:hover {
background: #000;
}
}
Partials
Partials allow you to split your Sass code into multiple files, making it easier to manage large projects.
// _base.scss
$font-stack: Helvetica, sans-serif;
$primary-color: #333;
body {
font: 100% $font-stack;
color: $primary-color;
}
// styles.scss
@use 'base';
.inverse {
background-color: base.$primary-color;
color: white;
}
Best Practices and Tools
Using Sourcemaps
Sourcemaps help you debug your CSS by mapping the compiled CSS back to the original Sass files. This is especially useful when working with complex stylesheets.
Choosing the Right Tools
There are several tools available to make working with Sass easier. Here are a few recommendations:
- Koala: A free, open-source app available for Mac, Windows, and Linux.
- CodeKit: A paid tool for Mac users that simplifies the compilation process.
- Gulp: A task runner that can automate the compilation of Sass files.
For hosting your WordPress site, consider using a reliable service like Kinsta, which offers optimized performance and security.
Real-World Examples and Case Studies
Using Sass in real-world projects can significantly streamline your development process. Here’s an example of how you might organize a WordPress theme using Sass:
wp-content/themes/your-theme/
├── sass/
│ ├── _variables.scss
│ ├── _mixins.scss
│ ├── _partials.scss
│ ├── style.scss
├── style.css
In this example, _variables.scss
contains all your variables, _mixins.scss
contains reusable mixins, and _partials.scss
contains partials for different sections of your site.
Conclusion and Next Steps
Using Sass in your WordPress development can make your workflow more efficient and your code more maintainable. Here are some key takeaways:
- Variables: Use variables to store reusable values.
- Nesting: Nest your CSS selectors for better readability.
- Mixins: Use mixins for repetitive styles.
- Partials: Split your code into manageable partials.
- Tools: Use tools like Koala, CodeKit, or Gulp to automate compilation.
If you’re looking to take your WordPress development to the next level, consider reaching out to Belov Digital Agency for expert guidance and support.
By integrating Sass into your workflow, you can write less CSS and achieve more, making your development process smoother and more efficient. For more tips and tricks on WordPress development, check out our other blog posts on Belov Digital Agency’s blog.