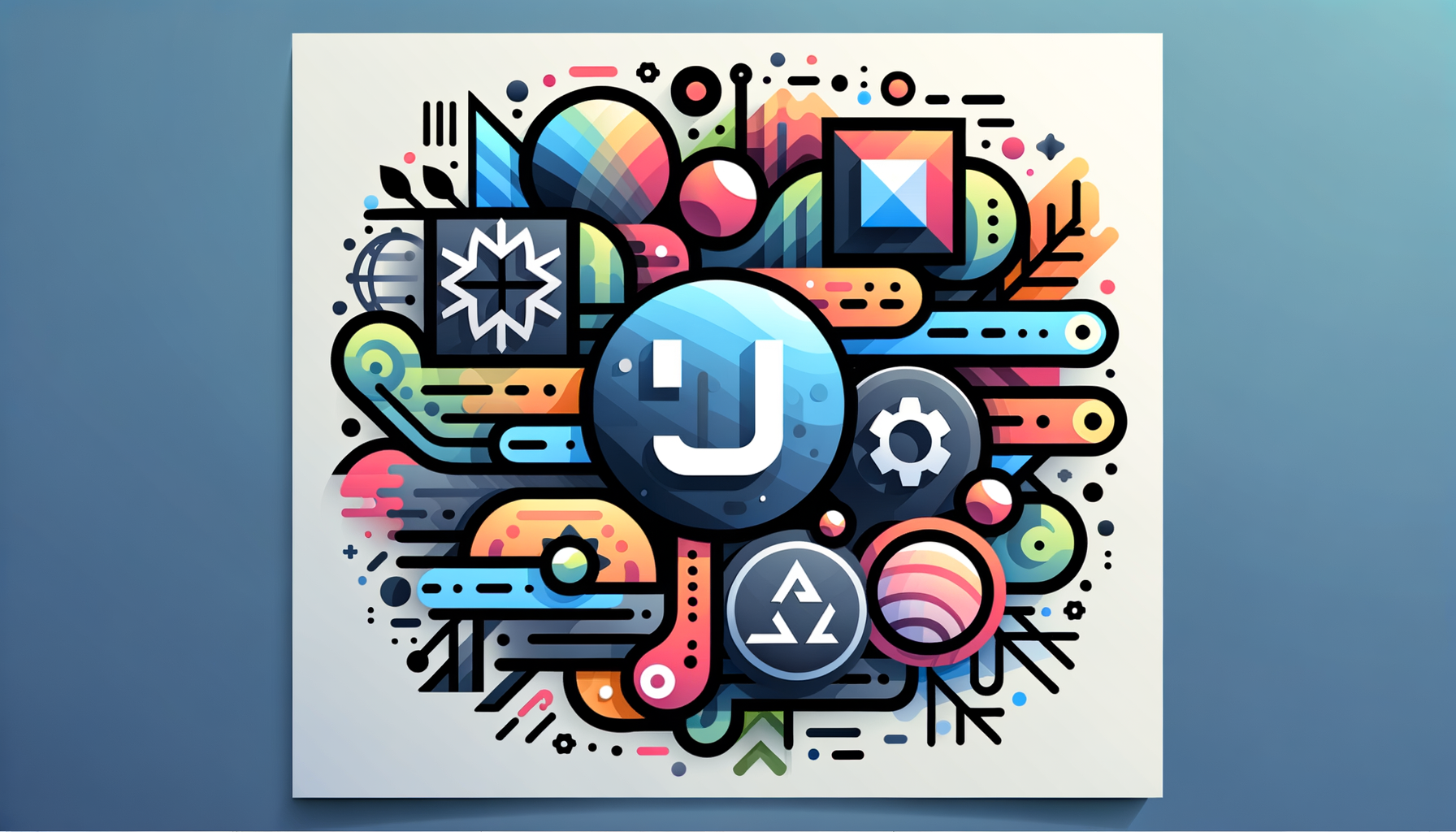
Enhancing WordPress Development with TypeScript: A Comprehensive Guide
Introduction to TypeScript and Its Benefits
In the realm of web development, particularly with WordPress, maintaining high code quality and reducing errors is crucial. This is where TypeScript comes into play, offering a robust solution by extending JavaScript with static typing and other advanced features. TypeScript, a superset of JavaScript, compiles to plain JavaScript and is supported by all browsers, making it an ideal choice for enhancing your WordPress development projects.
What is TypeScript?
TypeScript is JavaScript’s typed superset, designed to address the limitations of JavaScript’s dynamic typing. It adds features like type declarations, interfaces, and classes, which help in catching errors early and improving code maintainability. If you write valid JavaScript code, it is also valid TypeScript code, making the transition seamless.
Installing TypeScript for WordPress Development
To get started with TypeScript in your WordPress projects, you need to install it as a dev-dependency using npm. Here are the steps to follow:
- Initialize your package.json:
Follow the wizard to set up your package.json file.npm init
- Install TypeScript and necessary dependencies:
Alternatively, you can install all these packages at once:npm install typescript --save-dev npm install @wordpress/scripts --save-dev npm install ts-loader --save-dev
This setup includesnpm i -D ts-loader typescript @wordpress/scripts
@wordpress/scripts
which simplifies the process of bundling your JavaScript and SCSS files, andts-loader
for handling TypeScript files.
Setting Up Your Project Structure
For a smooth integration of TypeScript with your WordPress project, you need to set up the right file structure and configuration files.
- Create necessary files:
index.php
: The entry point for your plugin.tsconfig.json
: Configuration file for TypeScript.webpack.config.js
: Configuration file for Webpack, which is used by@wordpress/scripts
.
Here is an example of how your tsconfig.json
might look:
{
"compilerOptions": {
"outDir": "build",
"sourceMap": true,
"noImplicitAny": true,
"moduleResolution": "node",
"esModuleInterop": true,
"allowSyntheticDefaultImports": true
}
}
And here’s a basic webpack.config.js
to get you started:
const defaultConfig = require('@wordpress/scripts/config/webpack.config');
const path = require('path');
const fs = require('fs');
const glob = require('glob');
module.exports = env => {
return {
...defaultConfig,
module: {
...defaultConfig.module,
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/,
},
],
},
plugins: [
...defaultConfig.plugins,
{
apply: compiler => {
compiler.hooks.afterEmit.tap('rename', rename);
},
},
],
};
};
function rename() {
const blockJsonFiles = glob.sync(join(process.cwd(), 'build', '**', 'block.json'));
if (blockJsonFiles) {
blockJsonFiles.forEach(filePath => {
let blockJson = require(filePath);
// Update script and style paths
blockJson.editorScript = blockJson.editorScript.replace('.tsx', '.js');
blockJson.script = blockJson.script.replace('.tsx', '.js');
blockJson.editorStyle = blockJson.editorStyle.replace('.scss', '.css');
blockJson.style = blockJson.style.replace('.scss', '.css');
fs.writeFile(filePath, JSON.stringify(blockJson, null, 2), function writeJSON(error) {
if (error) {
return console.log(error);
}
});
});
}
}
This configuration ensures that your TypeScript files are compiled and bundled correctly.
Using TypeScript with the Block Editor
TypeScript integrates seamlessly with the WordPress Block Editor, especially when using the Interactivity API. Here’s how you can leverage TypeScript for building interactive blocks:
Inferring Types from Your Client Store Definition
When creating a store using the store
function, TypeScript can automatically infer the types of the store’s properties such as state
, actions
, and callbacks
. Here’s an example of a simple counter block:
// view.ts
const myStore = store('myCounterPlugin', {
state: {
counter: 0,
},
actions: {
increment() {
myStore.state.counter += 1;
},
},
callbacks: {
log() {
console.log(`counter: ${myStore.state.counter}`);
},
},
});
TypeScript will infer the types correctly, allowing you to write type-safe code without explicit type definitions.
Explicit Server Types and Multiple Store Parts
For more complex scenarios, you can explicitly define types for data defined on the server. This ensures that all parts of your store are correctly typed, even if your store is split into multiple parts. Here’s an example of how you can define explicit server types:
// server.ts
interface ServerState {
counter: number;
}
const initialServerState: ServerState = {
counter: 0,
};
// client.ts
const myStore = store('myCounterPlugin', {
state: initialServerState,
actions: {
increment() {
myStore.state.counter += 1;
},
},
});
This approach ensures that your server and client code are in sync and type-safe.
Real-World Examples and Case Studies
Building a Complex Block with TypeScript
Let’s consider a real-world example of building a complex block that includes multiple components, each with their own props and state. Using TypeScript, you can declare the types of your props and state variables, as well as the types of the data that your functions return. Here’s an example of how you might structure such a block:
// block.tsx
interface BlockProps {
title: string;
description: string;
}
const MyBlock: React.FC = ({ title, description }) => {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
{title}
{description}
Count: {count}
);
};
This example demonstrates how TypeScript helps in maintaining type safety and reducing errors in complex blocks.
Conclusion and Next Steps
Using TypeScript in your WordPress development projects can significantly enhance code quality, reduce bugs, and improve maintainability. Here are some key takeaways:
- Static Typing: TypeScript’s static typing helps catch errors at compile-time, reducing the likelihood of runtime errors.
- Better Code Completion: With type declarations, your IDE can provide better code completion suggestions, making development more efficient.
- Easier Refactoring: TypeScript’s type system makes refactoring easier by allowing you to rename symbols across multiple files with a single command.
If you’re looking to improve the reliability and maintainability of your WordPress projects, consider integrating TypeScript into your workflow. For more resources and guides, you can visit the Belov Digital Agency blog, where we regularly post articles on advanced WordPress development techniques.
Hosting Considerations
When hosting your WordPress site, it’s crucial to choose a reliable hosting provider that supports your development needs. Kinsta, for example, offers high-performance hosting solutions that are optimized for WordPress, making it an excellent choice for developers who value speed and reliability.
Getting Help and Support
If you have any questions or need further assistance with integrating TypeScript into your WordPress projects, don’t hesitate to Contact Us. Our team at Belov Digital Agency is here to help you navigate the complexities of modern web development.
By embracing TypeScript, you can take your WordPress development to the next level, ensuring that your projects are more robust, maintainable, and scalable. Happy coding.