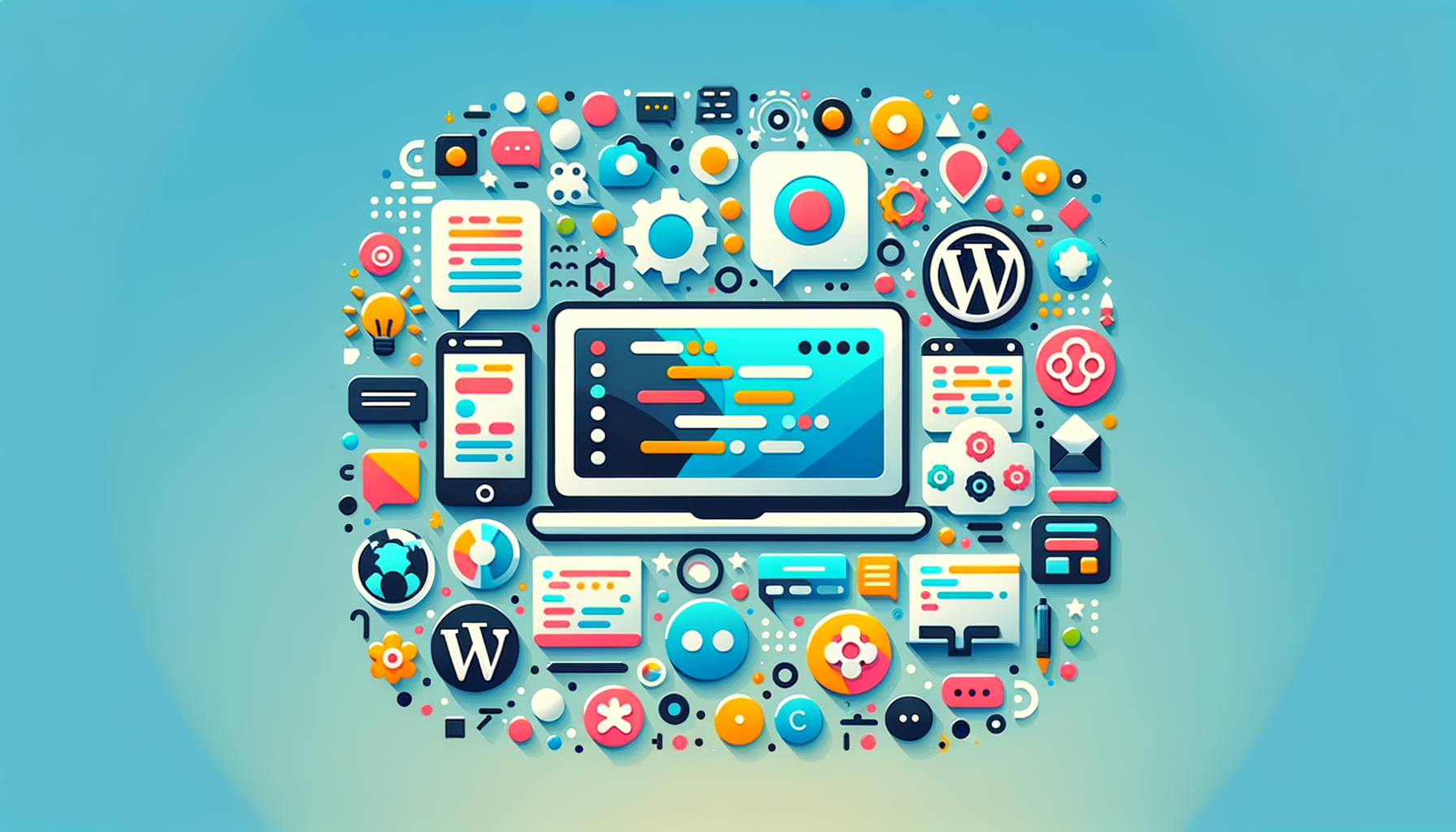
Leveraging Vue.js for Enhanced WordPress Development
In the ever-evolving landscape of web development, combining modern JavaScript frameworks with traditional content management systems (CMS) has become a powerful strategy for creating dynamic and interactive web experiences. One such combination that has gained significant traction is integrating Vue.js with WordPress. Here’s a comprehensive guide on how to harness the capabilities of Vue.js to enhance your WordPress development.
Why Choose Vue.js for WordPress Development?
Vue.js, introduced by Evan You in 2014, has rapidly gained popularity among web developers due to its ease of use, flexibility, and performance. Here are some key reasons why Vue.js is an excellent choice for WordPress development:
-
Ease of Learning and Use
Vue.js boasts a clear and concise syntax, making it accessible to developers of all experience levels. The extensive documentation, tutorials, and code examples available for Vue.js simplify the learning process, allowing developers to quickly get started with building interactive user interfaces.
-
Component-Based Architecture
Vue.js is built on a component-based development approach, which divides user interfaces into reusable and self-contained components. This architecture makes it easier to build and maintain complex web applications, as each component can be developed and tested independently before being integrated into the main application.
-
Optimized Performance
Vue.js is designed with performance in mind, ensuring that applications built with this framework are fast and efficient. Its lightweight architecture and intelligent reactivity system enable efficient updates to the user interface when the underlying data changes, providing a fluid and responsive user experience.
-
Vibrant Ecosystem
The Vue.js ecosystem is rich with community-developed plugins and tools, including routers, state managers, component libraries, and development tools. This ecosystem provides everything developers need to build modern, powerful web applications.
Setting Up Your Environment for Vue.js and WordPress
To integrate Vue.js into your WordPress project, you need to set up your development environment properly.
-
Node.js and npm
Ensure you have Node.js and npm installed, as these are required to install Vue.js and other necessary packages. You can download Node.js from the official Node.js website.
-
Webpack or Another Bundler
Use tools like Webpack to bundle your Vue.js components and other assets. You can set up a local WordPress environment using tools like Local by Flywheel or XAMPP.
Creating a Basic Vue.js Component
Here’s a step-by-step guide to creating a simple Vue.js component:
<template>
<div class="hello">
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello, World!'
}
}
}
</script>
<style>
.hello {
color: red;
}
</style>
This example creates a basic HelloWorld.vue
component that displays the message “Hello, World!” in red color.
Integrating Vue.js into a WordPress Theme
To integrate Vue.js into your WordPress theme, follow these steps:
-
Enqueue Vue.js
First, enqueue Vue.js in your theme’s
functions.php
file. You can use a CDN or bundle it with your assets.function enqueue_vue() { wp_enqueue_script('vue-js', 'https://cdn.jsdelivr.net/npm/vue@3/dist/vue.global.js', array(), null, true); } add_action('wp_enqueue_scripts', 'enqueue_vue');
-
Set Up the DOM Element
Ensure there is a DOM element in your WordPress page for the Vue app to mount on. You can create a custom page template or use an existing one.
<!-- templates/vue-search-app-template.php --> <div id="wp-vue-app"></div>
-
Register and Enqueue the Vue App
Register and enqueue your Vue app script in WordPress.
function enqueue_vue_app() { wp_enqueue_script('vue-app', get_template_directory_uri() . '/path/to/your/vue/app.js', array('vue-js'), null, true); } add_action('wp_enqueue_scripts', 'enqueue_vue_app');
-
Inform Vue About the Mount Point
Tell Vue where to mount the app by specifying the DOM element in your Vue app’s
main.js
file.import { createApp } from 'vue'; import App from './App.vue'; createApp(App).mount('#wp-vue-app');
Handling Data with the WordPress REST API
When integrating Vue.js with WordPress, you can leverage the WordPress REST API to handle data. Here’s an example of how to fetch data using Vue 3 and the WordPress REST API:
import { ref, onMounted } from 'vue';
export default {
setup() {
const posts = ref([]);
onMounted(async () => {
const response = await fetch('https://yourwebsite.com/wp-json/wp/v2/posts');
const data = await response.json();
posts.value = data;
});
return {
posts
};
}
};
This example fetches posts from the WordPress REST API and displays them in the Vue component.
Real-World Examples and Case Studies
Here are some examples of how Vue.js can be integrated with WordPress:
-
Single Page Search App
One practical example of integrating Vue.js with WordPress is building a Single Page Search App. This involves creating a Vue app that interacts with the WordPress REST API to fetch and display search results. You can follow the detailed guide on WPMU DEV to implement this.
-
Custom Themes and Plugins
Developers at Hike Branding have successfully integrated Vue.js into WordPress themes and plugins, creating highly interactive and dynamic web applications. Their approach involves setting up a local development environment, creating Vue components, and integrating these components into WordPress using shortcodes or widgets.
Best Practices and Tools
Here are some best practices and tools for integrating Vue.js with WordPress:
-
Using Vue-CLI
For a more streamlined development process, use the official Vue-CLI to scaffold your Vue project. This sets up a Vue project with Webpack and configures it with a development server and other modern build tools.
-
Hosting Considerations
When deploying your Vue.js and WordPress application, consider using a reliable hosting service like Kinsta, which offers optimized performance and security for WordPress sites.
Conclusion and Next Steps
Integrating Vue.js with WordPress can significantly enhance the interactivity and user experience of your website. By following the steps outlined in this guide, you can start leveraging the power of Vue.js in your WordPress projects.
- Get Started: Set up your development environment and create your first Vue.js component.
- Explore Resources: Check out the extensive documentation and community resources available for Vue.js.
- Seek Expertise: If you need professional assistance, consider reaching out to a WordPress development agency like Belov Digital Agency for customized solutions.
- Stay Updated: Keep an eye on the latest developments in the Vue.js ecosystem and WordPress community to stay ahead in your web development journey.
By combining the flexibility of WordPress with the interactive capabilities of Vue.js, you can create dynamic, responsive, and engaging web applications that meet the modern standards of web development. For more detailed guides and tutorials, you can also visit Belov Digital Agency’s blog or contact us for personalized support.
Comments