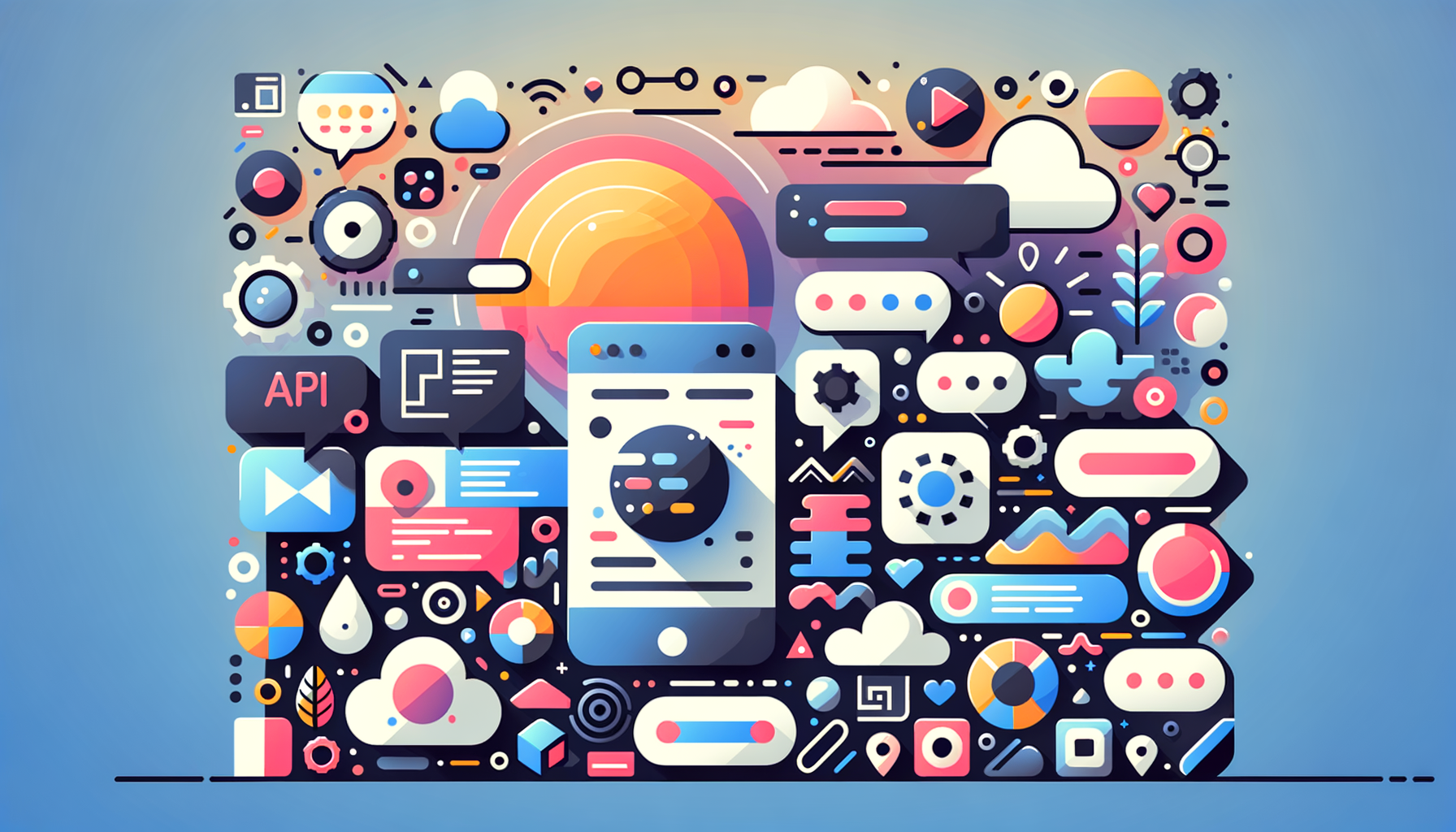
Leveraging the WordPress REST API for Modern Web Development
In the ever-evolving landscape of web development, the concept of headless websites has gained significant traction. This approach involves decoupling the frontend from the backend, allowing developers to utilize different technologies for each layer. At Belov Digital Agency, we specialize in harnessing the power of the WordPress REST API to build robust, flexible, and scalable headless websites.
Understanding Headless WordPress
Headless WordPress is a configuration where the traditional WordPress setup is modified to separate the content management system (CMS) from the frontend presentation layer. This decoupled architecture enables developers to use WordPress solely for content management while leveraging modern frontend frameworks like React, Angular, or Vue.js for the user interface.
Setting Up a Headless WordPress Environment
To get started with a headless WordPress setup, you need to ensure several technical prerequisites are in place.
Installing and Configuring WordPress
The first step is to install and configure WordPress. You can set up a new WordPress site either locally using tools like Local or DevKinsta, or on a server. It’s crucial to choose a blank theme to avoid unnecessary resource usage, as the frontend will be built separately.
Activating the WordPress REST API
The WordPress REST API is available by default in recent versions of WordPress. To verify its activation, navigate to http://yoursite.com/wp-json
in your browser. If you see JSON content, the API is working correctly. You can also enable the API by setting a pretty permalink structure under WordPress Settings → Permalinks.
Authentication and Security
Authentication is a critical aspect of interacting with the WordPress REST API. You can use basic authentication, OAuth, or JSON Web Tokens (JWT) for secure authentication. The JWT Auth plugin is particularly useful for implementing JWT authentication in WordPress, making it easy to manage tokens and secure your API endpoints.
Connecting to the WordPress REST API
To connect to the WordPress REST API, you need to send HTTP requests to the API endpoints. Here’s an example using JavaScript and the fetch
API with basic authentication:
const apiUrl = 'https://your-wordpress-site.com/wp-json/wp/v2/';
const username = 'your_username';
const password = 'your_password';
// Create a base64-encoded token from the username and password
const token = btoa(\`\${username}:\${password}\`);
// Fetch posts using basic authentication
fetch(apiUrl + 'posts', {
headers: {
Authorization: \`Basic \${token}\`
}
})
.then(response => response.json())
.then(data => {
console.log(data); // Process the retrieved data
})
.catch(error => {
console.error('Error fetching data:', error);
});
Building the Frontend
Once you can fetch data from the WordPress site, you can build the frontend using a framework of your choice. Here’s an example of creating a simple React app that displays WordPress post titles:
import React, { useState, useEffect } from 'react';
function App() {
const [posts, setPosts] = useState([]);
useEffect(() => {
fetch('https://your-wordpress-site.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(data => {
setPosts(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
return (
<div>
<h1>Headless WordPress App</h1>
<ul>
{posts.map(post => (
<li key={post.id}>{post.title.rendered}</li>
))}
</ul>
</div>
);
}
export default App;
Choosing the Right Frontend Framework
The choice of frontend framework is subjective and depends on your project’s specific needs, your team’s expertise, and the desired user experience. Popular choices include React, Angular, and Vue.js. For example, you can use React to build a dynamic and interactive frontend, or opt for a Static Site Generator (SSG) like Next.js or Gatsby and deploy it to hosting services like Kinsta’s Static Site Hosting for free.
Securing Your Headless WordPress Setup
Security is paramount in a headless WordPress setup, as you now have two systems to maintain. Ensure regular updates, backups, and the use of security plugins. The WordPress REST API includes functionality to verify connections, such as adding a check statement to the rest_authentication_errors
filter to require authentication for all requests.
Tools for Development and Testing
Several tools can enhance your development process when working with headless WordPress applications:
- MailHog: Useful for testing and debugging email functionality in local development environments.
- Insomnia: Aids in efficient API testing and response analysis.
- JWT Auth: Enables secure and reliable authentication using JSON Web Tokens.
Case Study: Building a Headless WordPress Application
A real-world example involves building a headless WordPress application that provides custom endpoints for an iOS app. This setup includes functionality for creating user accounts, getting user information, requesting tokens, and validating tokens. Tools like JWT Auth and Insomnia are crucial in this process for secure authentication and API testing.
Conclusion and Next Steps
The WordPress REST API offers a powerful way to build headless websites, providing the flexibility to use modern frontend frameworks while leveraging WordPress’s robust content management capabilities. By following the steps outlined above and utilizing the right tools, you can create dynamic, scalable, and secure headless WordPress applications.
If you’re looking to enhance your agency projects with headless setups, consider reaching out to Belov Digital Agency for expert guidance and implementation. Our team specializes in API development, decoupled architecture, and flexible frontend solutions to meet your project’s unique needs.